Tuesday, December 15, 2009
OpenScales
I've been working for some time now with the Openscales AS3 library. Finally we have a Flex GIS client. The library is work in progress, but already offers really nice features and functions. At some point soon I will share some of my work and code. I've been busy customising core functional elements including adding Yahoo and Bing baselayers, restricting WFS rendering to scale, allowing WFS layers to be turned on and off, custom styling the interface and components. More to come.
Labels:
client,
Flex,
maps. GIS,
open source,
OpenScales
Flex Web Services
I don't often use web services in Flex. But recetly found need to dip my toe in. The following I thought was an exellent simple example. taken from http://www.cflex.net/showFileDetails.cfm?ObjectID=582. Thanks Tracy.
<?xml version="1.0" encoding="utf-8"?>
<mx:Application xmlns:mx="http://www.adobe.com/2006/mxml" layout="absolute" xmlns="*"
creationComplete="getWeather()" >
<mx:Script>
<![CDATA[
import mx.controls.dataGridClasses.DataGridColumn;
import mx.rpc.events.ResultEvent;
import mx.managers.CursorManager;
import mx.controls.Alert;
default xml namespace = "http://www.webservicex.net"; //necessary to access the xml elements easily
[Bindable]private var _xmlResult:XML; //holds the result xml
[Bindable]private var _xlDayData:XMLList; //dataProvider for the day weather dataGrid
[Bindable]private var _sPlace:String;
/** invokes the web service operation to get the weather */
private function getWeather():void
{
CursorManager.setBusyCursor();
WS.GetWeatherByZipCode.send();
}
/** called by the WebService result event. Sets the dataProviders as necessary */
private function onResult(oEvent:ResultEvent):void
{
_xmlResult = XML(oEvent.result);
var xmlResultNode:XML = _xmlResult.GetWeatherByZipCodeResult[0];
var xmlDetailsNode:XML = xmlResultNode.Details[0];
outputInfo.text = _xmlResult.toXMLString();
_sPlace = xmlResultNode.PlaceName.text() + ", " + xmlResultNode.StateCode.text();
_xlDayData = xmlDetailsNode.WeatherData;
CursorManager.removeBusyCursor();
}//onResult
/** labelFunction for DataGrid. It seems that the namespace on the xml makes
* using the DataGridColumn dataField not work. At least I couldn't get it to work. */
private function lfDayData(oItem:Object, dgc:DataGridColumn):String
{
var sReturn:String = "";
var xmlItem:XML = XML(oItem); //get the item object cast as an xml object
var sHeaderText:String = dgc.headerText; //get the header text for this column
switch (sHeaderText) //logic to determine which node to get the data from
{
case "Day":
sReturn = xmlItem.Day.text();
break;
case "High":
sReturn = xmlItem.MaxTemperatureF.text();
break;
case "Low":
sReturn = xmlItem.MinTemperatureF.text();
break;
}
return sReturn;
}
]]>
</mx:Script>
<mx:WebService id="WS" wsdl="http://www.webservicex.net/WeatherForecast.asmx?WSDL"
useProxy="false"
fault="Alert.show(event.fault.faultString), 'Error'"
result="onResult(event)" >
<mx:operation name="GetWeatherByZipCode" resultFormat="e4x" >
<mx:request>
<ZipCode>{zipcode.text}</ZipCode>
</mx:request>
</mx:operation>
</mx:WebService>
<mx:Panel title="WebService Example" height="75%"
paddingTop="10" paddingBottom="10" paddingLeft="10" paddingRight="10" id="myPanel">
<mx:TextArea x="200" width="400" height="250" id="outputInfo" />
<mx:HBox>
<mx:Label width="100%" color="blue"
text="Enter a zip code."/>
<mx:TextInput id="zipcode" text="30117"/>
<mx:Button label="Get weather" click="getWeather()"/>
</mx:HBox>
<mx:Text id="txtPlace" htmlText="{_sPlace}"/>
<mx:DataGrid dataProvider="{_xlDayData}" height="180">
<mx:columns>
<mx:DataGridColumn labelFunction="lfDayData" headerText="Day" width="200" />
<mx:DataGridColumn labelFunction="lfDayData" headerText="High" width="100" />
<mx:DataGridColumn labelFunction="lfDayData" headerText="Low" width="100" />
</mx:columns>
</mx:DataGrid>
</mx:Panel>
</mx:Application>
<?xml version="1.0" encoding="utf-8"?>
<mx:Application xmlns:mx="http://www.adobe.com/2006/mxml" layout="absolute" xmlns="*"
creationComplete="getWeather()" >
<mx:Script>
<![CDATA[
import mx.controls.dataGridClasses.DataGridColumn;
import mx.rpc.events.ResultEvent;
import mx.managers.CursorManager;
import mx.controls.Alert;
default xml namespace = "http://www.webservicex.net"; //necessary to access the xml elements easily
[Bindable]private var _xmlResult:XML; //holds the result xml
[Bindable]private var _xlDayData:XMLList; //dataProvider for the day weather dataGrid
[Bindable]private var _sPlace:String;
/** invokes the web service operation to get the weather */
private function getWeather():void
{
CursorManager.setBusyCursor();
WS.GetWeatherByZipCode.send();
}
/** called by the WebService result event. Sets the dataProviders as necessary */
private function onResult(oEvent:ResultEvent):void
{
_xmlResult = XML(oEvent.result);
var xmlResultNode:XML = _xmlResult.GetWeatherByZipCodeResult[0];
var xmlDetailsNode:XML = xmlResultNode.Details[0];
outputInfo.text = _xmlResult.toXMLString();
_sPlace = xmlResultNode.PlaceName.text() + ", " + xmlResultNode.StateCode.text();
_xlDayData = xmlDetailsNode.WeatherData;
CursorManager.removeBusyCursor();
}//onResult
/** labelFunction for DataGrid. It seems that the namespace on the xml makes
* using the DataGridColumn dataField not work. At least I couldn't get it to work. */
private function lfDayData(oItem:Object, dgc:DataGridColumn):String
{
var sReturn:String = "";
var xmlItem:XML = XML(oItem); //get the item object cast as an xml object
var sHeaderText:String = dgc.headerText; //get the header text for this column
switch (sHeaderText) //logic to determine which node to get the data from
{
case "Day":
sReturn = xmlItem.Day.text();
break;
case "High":
sReturn = xmlItem.MaxTemperatureF.text();
break;
case "Low":
sReturn = xmlItem.MinTemperatureF.text();
break;
}
return sReturn;
}
]]>
</mx:Script>
<mx:WebService id="WS" wsdl="http://www.webservicex.net/WeatherForecast.asmx?WSDL"
useProxy="false"
fault="Alert.show(event.fault.faultString), 'Error'"
result="onResult(event)" >
<mx:operation name="GetWeatherByZipCode" resultFormat="e4x" >
<mx:request>
<ZipCode>{zipcode.text}</ZipCode>
</mx:request>
</mx:operation>
</mx:WebService>
<mx:Panel title="WebService Example" height="75%"
paddingTop="10" paddingBottom="10" paddingLeft="10" paddingRight="10" id="myPanel">
<mx:TextArea x="200" width="400" height="250" id="outputInfo" />
<mx:HBox>
<mx:Label width="100%" color="blue"
text="Enter a zip code."/>
<mx:TextInput id="zipcode" text="30117"/>
<mx:Button label="Get weather" click="getWeather()"/>
</mx:HBox>
<mx:Text id="txtPlace" htmlText="{_sPlace}"/>
<mx:DataGrid dataProvider="{_xlDayData}" height="180">
<mx:columns>
<mx:DataGridColumn labelFunction="lfDayData" headerText="Day" width="200" />
<mx:DataGridColumn labelFunction="lfDayData" headerText="High" width="100" />
<mx:DataGridColumn labelFunction="lfDayData" headerText="Low" width="100" />
</mx:columns>
</mx:DataGrid>
</mx:Panel>
</mx:Application>
Sunday, November 22, 2009
Flex and SOAP
Its always fun to work with Web service in Flex. Those pesky headers! Found a couple of terrific links on the subject. Now its easy :-)
http://www.actionscript.org/forums/showthread.php3?t=195916&highlight=e4x+faq
http://craigkaminsky.blogspot.com/2009/05/flex-3-make-net-namespaces-in-soap-xml.html
Just to put some code behind this....
Here is part of the feed:
<?xml version="1.0" encoding="utf-8" standalone="yes" ?>
<feed xml:base="http://www.placespr.com/WebDataService1.svc/" xmlns:d="http://schemas.microsoft.com/ado/2007/08/dataservices" xmlns:m="http://schemas.microsoft.com/ado/2007/08/dataservices/metadata" xmlns="http://www.w3.org/2005/Atom">
<title type="text">ptg_categories</title>
<id>http://www.placespr.com/webdataservice1.svc/ptg_categories</id>
<updated>2009-11-22T15:26:36Z</updated>
<link rel="self" title="ptg_categories" href="ptg_categories" />
<entry>
<id>http://www.placespr.com/WebDataService1.svc/ptg_categories(1)</id>
<title type="text" />
<updated>2009-11-22T15:26:36Z</updated>
<author>
<name />
</author>
<link rel="edit" title="ptg_categories" href="ptg_categories(1)" />
<category term="ponce_1000Model.ptg_categories" scheme="http://schemas.microsoft.com/ado/2007/08/dataservices/scheme" />
<content type="application/xml">
<m:properties>
<d:cat_id m:type="Edm.Int32">1</d:cat_id>
<d:name m:null="true" />
<d:service>hotels.asmx</d:service>
<d:icon>/images/icons/hotels.gif</d:icon>
<d:ordering m:type="Edm.Int32">1</d:ordering>
<d:counting m:type="Edm.Int32">75</d:counting>
</m:properties>
</content>
</entry>
......
and here is the modified code from these excellent links:
private function getCategoriesResult(evt:ResultEvent):void {
var respXML:XML = evt.result as XML;
var xmlSource:String = respXML.toXMLString();
//Strip off the headers
xmlSource = xmlSource.replace(/<[^!?]?[^>]+?>/g, removeNamspaces); // will invoke the function detailed below
var cleanXML:XML = XML(xmlSource);
for each( var item:XML in cleanXML.entry ) // looping over the reportHistory elements to act on them
{
trace( "name: " + item.content.properties.service);
}
}
// Web service response cleaner function
private function removeNamspaces(...rest):String
{
rest[0] = rest[0].replace(/xmlns[^"]+\"[^"]+\"/g, "");
var attrs:Array = rest[0].match(/\"[^"]*\"/g);
rest[0] = rest[0].replace(/\"[^"]*\"/g, "%attribute value%");
rest[0] = rest[0].replace(/(<\/?|\s)\w+\:/g, "$1");
while (rest[0].indexOf("%attribute value%") > 0)
{
rest[0] = rest[0].replace("%attribute value%", attrs.shift());
}
return rest[0];
}
http://www.actionscript.org/forums/showthread.php3?t=195916&highlight=e4x+faq
http://craigkaminsky.blogspot.com/2009/05/flex-3-make-net-namespaces-in-soap-xml.html
Just to put some code behind this....
Here is part of the feed:
<?xml version="1.0" encoding="utf-8" standalone="yes" ?>
<feed xml:base="http://www.placespr.com/WebDataService1.svc/" xmlns:d="http://schemas.microsoft.com/ado/2007/08/dataservices" xmlns:m="http://schemas.microsoft.com/ado/2007/08/dataservices/metadata" xmlns="http://www.w3.org/2005/Atom">
<title type="text">ptg_categories</title>
<id>http://www.placespr.com/webdataservice1.svc/ptg_categories</id>
<updated>2009-11-22T15:26:36Z</updated>
<link rel="self" title="ptg_categories" href="ptg_categories" />
<entry>
<id>http://www.placespr.com/WebDataService1.svc/ptg_categories(1)</id>
<title type="text" />
<updated>2009-11-22T15:26:36Z</updated>
<author>
<name />
</author>
<link rel="edit" title="ptg_categories" href="ptg_categories(1)" />
<category term="ponce_1000Model.ptg_categories" scheme="http://schemas.microsoft.com/ado/2007/08/dataservices/scheme" />
<content type="application/xml">
<m:properties>
<d:cat_id m:type="Edm.Int32">1</d:cat_id>
<d:name m:null="true" />
<d:service>hotels.asmx</d:service>
<d:icon>/images/icons/hotels.gif</d:icon>
<d:ordering m:type="Edm.Int32">1</d:ordering>
<d:counting m:type="Edm.Int32">75</d:counting>
</m:properties>
</content>
</entry>
......
and here is the modified code from these excellent links:
private function getCategoriesResult(evt:ResultEvent):void {
var respXML:XML = evt.result as XML;
var xmlSource:String = respXML.toXMLString();
//Strip off the headers
xmlSource = xmlSource.replace(/<[^!?]?[^>]+?>/g, removeNamspaces); // will invoke the function detailed below
var cleanXML:XML = XML(xmlSource);
for each( var item:XML in cleanXML.entry ) // looping over the reportHistory elements to act on them
{
trace( "name: " + item.content.properties.service);
}
}
// Web service response cleaner function
private function removeNamspaces(...rest):String
{
rest[0] = rest[0].replace(/xmlns[^"]+\"[^"]+\"/g, "");
var attrs:Array = rest[0].match(/\"[^"]*\"/g);
rest[0] = rest[0].replace(/\"[^"]*\"/g, "%attribute value%");
rest[0] = rest[0].replace(/(<\/?|\s)\w+\:/g, "$1");
while (rest[0].indexOf("%attribute value%") > 0)
{
rest[0] = rest[0].replace("%attribute value%", attrs.shift());
}
return rest[0];
}
Labels:
ESRI arcmap flex api,
headers,
web service,
XML
Tuesday, November 17, 2009
IE8 Vista Problem
I'll not br rotten about Microsoft, since I develop on Windows .. but sometimes!!
I found I could not open IE, out of the blue. Spent a frustrating couple of hours troubleshooting. All to do with security updates and IE settins. The solution which worked of me is as follows:
Open internet explorer and go to menu TOOLS, than to INTERNET OPTIONS. Open the CONNECTION tab,and click on SETTINGS. You then uncheck the 'use of proxy server for this connection' and click ok .
I found I could not open IE, out of the blue. Spent a frustrating couple of hours troubleshooting. All to do with security updates and IE settins. The solution which worked of me is as follows:
Open internet explorer and go to menu TOOLS, than to INTERNET OPTIONS. Open the CONNECTION tab,and click on SETTINGS. You then uncheck the 'use of proxy server for this connection' and click ok .
Sunday, November 15, 2009
AlivePDF
Its been a hectic time with project work. Much to share particularly with regards the ArcGIS Flex API and the open source OpenScales Flex library.
Just a quick mention today on AlivePDF. Historically, I've had to generate PDF's in PHP. AlivePDF is based on the PHP library FPDF. It makes generating PDF's from Flex very straighforward. Alex Britez has written a simple example here.
Just a quick mention today on AlivePDF. Historically, I've had to generate PDF's in PHP. AlivePDF is based on the PHP library FPDF. It makes generating PDF's from Flex very straighforward. Alex Britez has written a simple example here.
Labels:
AlivePDF,
ArcGIS API,
Flex,
maps,
OpenScales
Saturday, October 17, 2009
Open Source GIS Clients
I have had a number of companies recently approach me about open source GIS options. This has something to do with my recently published articles. One interesting area has been an investigation into Flex GIS clients. Openlayers is the premier Web 2.0 GIS client; well supported, a rich library filled with an array of functionality .. but written in Javascript. Now I have nothing against Javascript, its free, has a big community behind it and runs in the browser. But I'm a Flex developer. I prefer it because .. it has an excellent IDE which makes for easy debugging, developing sophisticated interactive application is both quick and easy, applications run the same in all browsers, actionscript is object oriented. Actionscript is just nicer and easier to work with .. in my view.
So enough. Anybody who argues against these opinions I would not oppose. Its just how I feel about it. Anyway, the whole Flex GIS open source client issue. I have worked with Modest Maps for sometime now to develop mostly consumer maps. Stop right there! Consumer maps? The world of online maps remains somewhat split. GIS maps and consumer maps. Consumer maps I define as the use of basemaps - tiles which are derived from satellite images or vector road maps for example - to build mash ups; video, imagery, text using, often, the addition of map markers for access (or clickable icons geospatially placed on the map). Here is a simple example. Google, Yahoo, Bing, mapquest offer largely API's for developing consumer maps.
So what are online GIS maps. A simple question? Well maybe. GIS has moved from the desktop to the Web. It was very much a niche market. Geography type nerds generating applications and analyses in large part for government (a generalisation but close to reality). The frustration for GIS vendors was how do they broaden the market. ESRI and the other key private vendors struggled to sell into the enterprise. They still do in fact.
Suddenly google maps arrived on the scene. It shook the mapping industry to its core. Internet maps were everywhere. Its worth noting that Mapquest preceded Google, but the fanfare (and $) waited for Googles arrival. Suddenly the other GIS vendors needed to react. ESRI produced rival online products. But with a GIS twist.
So here we are today. A plethora of consumer map APIs. And ESRI sitting in a somewhat awkward position offering free API's hooking easily into their own server product(s) ArcGIS (not so easily into other providers). Offering consumer and GIS API functionality. With a focus to sell their complete stack to those interested. Their purpose is to make money.
So how about those of us wanting to build online GIS maps, but avoid the high cost of the private vendor solutions? The open source GIS stack is very mature. But how about Flex clients?
I've spent some time looking at the options. Base requirements include:
- Rendering both WFS and WMS in combination
- Overlays of these services on various basemaps
- Drawing tools for line point and polygons
- Identify functionality
I looked at both Modest Maps and OpenScales. Though not its focus there has been some work done on Modest Maps toward some level of interaction with spatial servers. I added a WMS layer at:
http://www.flexmappers.com/mapserving/mapservingWMS/
Note, I had to make some tweeks to the WMS provider, provided with the library.
Openscales is less mature. But very impressive for how long development has been underway. I built the following map application:
http://www.flexmappers.com/mapserving/openscales.html
It supplies much of the base functionality I was looking to include. WMS is proving a little challenging (but i suspect a style tag is mssing from the tile request) and the map interaction is not a smooth as Modest maps. Only openstreetmap is provided, but I am looking to port code from Modest maps to add this functionality.
Ultimately Flex should simply be rendering the points, lines, and polygons supplied by the server.
I am interested to see how easy it might be to port from one library to the other. Either way, Openscales is a very interesting project. One well worth supporting.
So enough. Anybody who argues against these opinions I would not oppose. Its just how I feel about it. Anyway, the whole Flex GIS open source client issue. I have worked with Modest Maps for sometime now to develop mostly consumer maps. Stop right there! Consumer maps? The world of online maps remains somewhat split. GIS maps and consumer maps. Consumer maps I define as the use of basemaps - tiles which are derived from satellite images or vector road maps for example - to build mash ups; video, imagery, text using, often, the addition of map markers for access (or clickable icons geospatially placed on the map). Here is a simple example. Google, Yahoo, Bing, mapquest offer largely API's for developing consumer maps.
So what are online GIS maps. A simple question? Well maybe. GIS has moved from the desktop to the Web. It was very much a niche market. Geography type nerds generating applications and analyses in large part for government (a generalisation but close to reality). The frustration for GIS vendors was how do they broaden the market. ESRI and the other key private vendors struggled to sell into the enterprise. They still do in fact.
Suddenly google maps arrived on the scene. It shook the mapping industry to its core. Internet maps were everywhere. Its worth noting that Mapquest preceded Google, but the fanfare (and $) waited for Googles arrival. Suddenly the other GIS vendors needed to react. ESRI produced rival online products. But with a GIS twist.
So here we are today. A plethora of consumer map APIs. And ESRI sitting in a somewhat awkward position offering free API's hooking easily into their own server product(s) ArcGIS (not so easily into other providers). Offering consumer and GIS API functionality. With a focus to sell their complete stack to those interested. Their purpose is to make money.
So how about those of us wanting to build online GIS maps, but avoid the high cost of the private vendor solutions? The open source GIS stack is very mature. But how about Flex clients?
I've spent some time looking at the options. Base requirements include:
- Rendering both WFS and WMS in combination
- Overlays of these services on various basemaps
- Drawing tools for line point and polygons
- Identify functionality
I looked at both Modest Maps and OpenScales. Though not its focus there has been some work done on Modest Maps toward some level of interaction with spatial servers. I added a WMS layer at:
http://www.flexmappers.com/mapserving/mapservingWMS/
Note, I had to make some tweeks to the WMS provider, provided with the library.
Openscales is less mature. But very impressive for how long development has been underway. I built the following map application:
http://www.flexmappers.com/mapserving/openscales.html
It supplies much of the base functionality I was looking to include. WMS is proving a little challenging (but i suspect a style tag is mssing from the tile request) and the map interaction is not a smooth as Modest maps. Only openstreetmap is provided, but I am looking to port code from Modest maps to add this functionality.
Ultimately Flex should simply be rendering the points, lines, and polygons supplied by the server.
I am interested to see how easy it might be to port from one library to the other. Either way, Openscales is a very interesting project. One well worth supporting.
Labels:
ESRI arcmap flex api,
GIS,
Modest Maps,
open source,
OpenLayers,
OpenScales
Sunday, October 11, 2009
Flex and PHP POST
I always forget the syntax when trying to get around the Flex sandbox and use PHP. On the Flex side we need the following:
[Bindable]private var pr_url:String;
..
private function init():void
{
..
pr_url = "url to SOAP service for example";
pr.send();
}
..
<mx:HTTPService id="pr" resultFormat="e4x" result="getMonths_result(event);" fault="getMonths_fault(event);" method="POST" url="http://www.flexmappers.com/puertorico/test.php">
<mx:request xmlns="">
<url>
{pr_url}
</url>
</mx:request>
</mx:HTTPService>
On the PHP side:
<?php
header('Content-Type: text/xml');
$url = $_POST["url"];
$data = file_get_contents($url);
echo $data;
?>
[Bindable]private var pr_url:String;
..
private function init():void
{
..
pr_url = "url to SOAP service for example";
pr.send();
}
..
<mx:HTTPService id="pr" resultFormat="e4x" result="getMonths_result(event);" fault="getMonths_fault(event);" method="POST" url="http://www.flexmappers.com/puertorico/test.php">
<mx:request xmlns="">
<url>
{pr_url}
</url>
</mx:request>
</mx:HTTPService>
On the PHP side:
<?php
header('Content-Type: text/xml');
$url = $_POST["url"];
$data = file_get_contents($url);
echo $data;
?>
Tuesday, September 22, 2009
OpenScales
While becoming increasingly more impressed with Openlayers I came across OpenScales. A porting of sorts of Openlayers to Actionscript 3.0. Some key links below:
My OpenStreetmap, WMS, WFS Test
http://www.flexmappers.com/openscales
Code Examples
http://openscales.org/svn/openscales/trunk/openscales-fx-examples/src/main/flex/
Demo
http://openscales.org/openscales.swf
api
http://openscales.org/mvn-site/
http://openscales.org/mvn-site/openscales-core/asdoc/index.html
GetCapabilities Test
http://openscales.org/proxy.php?url=http://sigma.openplans.org/geoserver/ows?service=WFS%26request%3DGetCapabilities
My OpenStreetmap, WMS, WFS Test
http://www.flexmappers.com/openscales
Code Examples
http://openscales.org/svn/openscales/trunk/openscales-fx-examples/src/main/flex/
Demo
http://openscales.org/openscales.swf
api
http://openscales.org/mvn-site/
http://openscales.org/mvn-site/openscales-core/asdoc/index.html
GetCapabilities Test
http://openscales.org/proxy.php?url=http://sigma.openplans.org/geoserver/ows?service=WFS%26request%3DGetCapabilities
Sunday, September 20, 2009
PostGIS
Here is an excellent guide to working with PostGIS. Some of my additional notes:
Load Shapefile to PostGIS
a) DB Connect:
psql -d gisdb -h localhost -U matt
b) Generate sql file from shapefile:
C:\Program files\PostgreSQL\8.2\bin>shp2pgsql.exe data\states > states.sql
c) Use pgAdmin to ru sql (tools -> query tool. In query tool open->go to the states.sql
Paul Ramsey wrote PostGIS. I recently shared some emails with him. Thought part of the discussion worth reprinting:
Q. What is the difference between PostGIS and ArcSDE?
A. At a very high level (or perhaps this is a very low level), PostGIS /
PostgreSQL is a database, and ArcSDE is not. ArcSDE is a "spatial
middleware", it runs as a separate process and talks to the real
database below it. PostGIS is a "dynamically loadable runtime" inside
the PostgreSQL process -- it runs inside the same address space as
PostgreSQL, from the point of view of the computer, it's the all the
same program.
PostGIS should be viewed in exactly the same way relative to
PostgreSQL as Oracle Spatial should vis-a-vis Oracle, SQL Server
Spatial vis-a-vis SQL Server, Informix Spatial Extender vis-a-vis
Informix, etc, etc, etc. It works exactly the same way as all those
other in-process spatial type extensions to relational databases. So,
call it an "extension", a "spatial type", a "data blade", etc.
Unfortunately, years of marketing by various vendors have muddied the
terminological water surrounding type extension mechanisms.
The most important functional differences with SDE have nothing to do
with spatial database functionality and everything to do with ESRI
product placement and marketing. You'll find that ESRI products talk
more directly to ArcSDE than they do to PostGIS (or Oracle Spatial or
SQL Server Spatial, for that matter) because ESRI is interested in
selling their whole stack (desktop + server + web apps) to their
clients, not just the ArcGIS component. It's a non-trivial benefit for a customer that (a) already has a lot of ESRI software and (b) can afford the maintenance overhead in dollars, time and expertise that ArcSDE extracts.
Load Shapefile to PostGIS
a) DB Connect:
psql -d gisdb -h localhost -U matt
b) Generate sql file from shapefile:
C:\Program files\PostgreSQL\8.2\bin>shp2pgsql.exe data\states > states.sql
c) Use pgAdmin to ru sql (tools -> query tool. In query tool open->go to the states.sql
Paul Ramsey wrote PostGIS. I recently shared some emails with him. Thought part of the discussion worth reprinting:
Q. What is the difference between PostGIS and ArcSDE?
A. At a very high level (or perhaps this is a very low level), PostGIS /
PostgreSQL is a database, and ArcSDE is not. ArcSDE is a "spatial
middleware", it runs as a separate process and talks to the real
database below it. PostGIS is a "dynamically loadable runtime" inside
the PostgreSQL process -- it runs inside the same address space as
PostgreSQL, from the point of view of the computer, it's the all the
same program.
PostGIS should be viewed in exactly the same way relative to
PostgreSQL as Oracle Spatial should vis-a-vis Oracle, SQL Server
Spatial vis-a-vis SQL Server, Informix Spatial Extender vis-a-vis
Informix, etc, etc, etc. It works exactly the same way as all those
other in-process spatial type extensions to relational databases. So,
call it an "extension", a "spatial type", a "data blade", etc.
Unfortunately, years of marketing by various vendors have muddied the
terminological water surrounding type extension mechanisms.
The most important functional differences with SDE have nothing to do
with spatial database functionality and everything to do with ESRI
product placement and marketing. You'll find that ESRI products talk
more directly to ArcSDE than they do to PostGIS (or Oracle Spatial or
SQL Server Spatial, for that matter) because ESRI is interested in
selling their whole stack (desktop + server + web apps) to their
clients, not just the ArcGIS component. It's a non-trivial benefit for a customer that (a) already has a lot of ESRI software and (b) can afford the maintenance overhead in dollars, time and expertise that ArcSDE extracts.
GeoDjango
Wikipedia defines Django as follows:
"Django's primary goal is to ease the creation of complex, database-driven websites. Django emphasizes reusability and "pluggability" of components, rapid development, and the principle of DRY (Don't Repeat Yourself). Python is used throughout, even for settings, files, and data models."
The two key links for GeoDjango:
GeoDjango Online Docs
Django Docs
"Django's primary goal is to ease the creation of complex, database-driven websites. Django emphasizes reusability and "pluggability" of components, rapid development, and the principle of DRY (Don't Repeat Yourself). Python is used throughout, even for settings, files, and data models."
The two key links for GeoDjango:
GeoDjango Online Docs
Django Docs
WMS and Bing/Yahoo Basemap Overlays
Here is an interesting issue I found today with WMS overlay. When WMS layers are created in either Mapserver or Geoserver then added as overlays to Yahoo or Bing their alignment is wrong.
Let me step back. As mentioned in earlier posts. One can create a WMS layer in geoserver and through the excellent config interface add this as a layer to Google earth.

All align perfectly. Now adding the same WMS to Modest Maps and overlaying it on Yahoo shows the WMS misaligned:

Turning to Openlayers, I find the same problem:

In each of the latter cases the WMS layer also looks stretched. I'll find the fix and post it.
Let me step back. As mentioned in earlier posts. One can create a WMS layer in geoserver and through the excellent config interface add this as a layer to Google earth.

All align perfectly. Now adding the same WMS to Modest Maps and overlaying it on Yahoo shows the WMS misaligned:

Turning to Openlayers, I find the same problem:

In each of the latter cases the WMS layer also looks stretched. I'll find the fix and post it.
Saturday, September 19, 2009
Map Technology Overview
I've been on a technology review of late. Mostly driven by client requests. I never cease to be amazed by what is now available. So far my thoughts in brief:
Mapserver is a pain to configure. Manually writing mapfiles, takes me back to the days of ArcIMS and axl files. Geoserver is far easier in every respect. It has a terrific admin interface. Setting up basic map services, WMS, WFS is easy. Exporting in formats read by google maps etc is also a piece of cake. If it were not for the need of a full application server, the choice of geospatial server would be easy. Geoserver also allows for easy generation of Georss, KML and viewing of map layers in openlayers.
Caching and tiling is another area which favours Geoserver. Geowebcache is integrated into geoserver. No need for additional work. The config screen allows for easy seeding. Mapserver works with tilecache. From the work I have done so far you tile mapserver by calling tilecache and passing the mapserver url.
I'm still working with openlayers but am so far very impressed. One thing I already notice is how much is there. I really like the Modest Maps Flex library. But with a smaller community, it does not have the same depth of code. I'm still playing here, so more feedback later.
I'd like to know one of the big fours - mapquest, MSN, Yahoo and Google - API's. I lean towards mapquest, largely due to the feedback I have read and my impressions from conferences. I recently signed up for their developer account. A couple of people were soon emailing me with contact info. On one side I thought this great, since support might be more forthcoming. On the other side, money reared its ugly head. Use of this service beyond local testing has a price tag! Reminds me why I so favour open source.
Other areas on my radar at the moment beyond testing the mapquest API include:
- Geodjango testing
- PostGIS and shapefile write up
- Javascript to Flex/Flash communication
I'll write more on this in due course. I asked Paul Ramsey (OpenGeo and PostGIS author) his thoughts on Geoserver versus Mapserver. Here is his repy:
If you are deep into OGC standards, Geoserver has a big advantage in
completeness of implementations of things like SLD and WFS. (Less on
WMS).
I did a talk last year which, though tongue-in-cheek addresses the
more important differences. Which are philosophical more than anything
else. Scriptable web rendering engine vs complete spatial web
environment.
http://s3.cleverelephant.ca/geoweb-mapserver.pdf
Most of the speed differences are gone at this point, they perform
very comparably for common use cases.
Mapserver is a pain to configure. Manually writing mapfiles, takes me back to the days of ArcIMS and axl files. Geoserver is far easier in every respect. It has a terrific admin interface. Setting up basic map services, WMS, WFS is easy. Exporting in formats read by google maps etc is also a piece of cake. If it were not for the need of a full application server, the choice of geospatial server would be easy. Geoserver also allows for easy generation of Georss, KML and viewing of map layers in openlayers.
Caching and tiling is another area which favours Geoserver. Geowebcache is integrated into geoserver. No need for additional work. The config screen allows for easy seeding. Mapserver works with tilecache. From the work I have done so far you tile mapserver by calling tilecache and passing the mapserver url.
I'm still working with openlayers but am so far very impressed. One thing I already notice is how much is there. I really like the Modest Maps Flex library. But with a smaller community, it does not have the same depth of code. I'm still playing here, so more feedback later.
I'd like to know one of the big fours - mapquest, MSN, Yahoo and Google - API's. I lean towards mapquest, largely due to the feedback I have read and my impressions from conferences. I recently signed up for their developer account. A couple of people were soon emailing me with contact info. On one side I thought this great, since support might be more forthcoming. On the other side, money reared its ugly head. Use of this service beyond local testing has a price tag! Reminds me why I so favour open source.
Other areas on my radar at the moment beyond testing the mapquest API include:
- Geodjango testing
- PostGIS and shapefile write up
- Javascript to Flex/Flash communication
I'll write more on this in due course. I asked Paul Ramsey (OpenGeo and PostGIS author) his thoughts on Geoserver versus Mapserver. Here is his repy:
If you are deep into OGC standards, Geoserver has a big advantage in
completeness of implementations of things like SLD and WFS. (Less on
WMS).
I did a talk last year which, though tongue-in-cheek addresses the
more important differences. Which are philosophical more than anything
else. Scriptable web rendering engine vs complete spatial web
environment.
http://s3.cleverelephant.ca/geoweb-mapserver.pdf
Most of the speed differences are gone at this point, they perform
very comparably for common use cases.
Labels:
ArcIMS,
ESRI,
flex api,
Geodjango,
geoserver,
Geowebcache,
mapquest Flash API,
mapserver,
Modest Maps,
PostGIS
Openlayers
The openlayers community seems to be becoming ever larger. Though I'm predominantly a Flex developer, its time I checked out Openlayers. On my recent WMS kick, I know combining WMS with other basemaps is well supported. Looking at the online docs I found this terrific examples page.
As a newbie I took out a few code examples:
1) Basic map
<html>
<head>
<title>OpenLayers Example</title>
<script
src="http://openlayers.org/api/OpenLayers.js"></script>
</head>
<body>
<div style="width:100%; height:100%" id="map"></div>
<script defer="defer" type="text/javascript">
var map = new OpenLayers.Map('map');
var wms = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://labs.metacarta.com/wms/vmap0", {layers: 'basic'} );
map.addLayer(wms);
map.zoomToMaxExtent();
</script>
</body>
</html>
2) WMS map
<html>
<head>
<title>OpenLayers Example</title>
<script
src="http://openlayers.org/api/OpenLayers.js"></script>
</head>
<body>
<div style="width:100%; height:100%" id="map"></div>
<script defer="defer" type="text/javascript">
var map = new OpenLayers.Map('map');
var wms = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://labs.metacarta.com/wms/vmap0", {layers: 'basic'} );
map.addLayer(wms);
map.zoomToMaxExtent();
</script>
</body>
</html>
3) Locally running WMS
<html xmlns="http://www.w3.org/1999/xhtml">
<script
src="http://openlayers.org/api/OpenLayers.js"></script>
</head>
<body>
<div style="width:100%; height:100%" id="map"></div>
<script defer="defer" type="text/javascript">
var map = new OpenLayers.Map('map');
var wms = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://localhost:8080/geoserver/wms", {layers: 'topp:states_ugl'} );
map.addLayer(wms);
map.zoomToMaxExtent();
</script>
</html>
4) Local WMS with Bing (note you will need to copy the OpenLayers.js file from
http://openlayers.org/dev/OpenLayers.js)
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>OpenLayers Bing Example</title>
<link rel="stylesheet" href="../theme/default/style.css" type="text/css" />
<link rel="stylesheet" href="style.css" type="text/css" />
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<script src="http://ecn.dev.virtualearth.net/mapcontrol/mapcontrol.ashx?v=6.2&mkt=en-us"></script>
<script src="OpenLayers.js"></script>
<script>
var map;
function init(){
map = new OpenLayers.Map("map");
map.addControl(new OpenLayers.Control.LayerSwitcher());
var shaded = new OpenLayers.Layer.VirtualEarth("Shaded", {
type: VEMapStyle.Shaded
});
var hybrid = new OpenLayers.Layer.VirtualEarth("Hybrid", {
type: VEMapStyle.Hybrid
});
var aerial = new OpenLayers.Layer.VirtualEarth("Aerial", {
type: VEMapStyle.Aerial
});
var wms = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://localhost:8080/geoserver/wms", {layers: 'topp:states_ugl'},
{
'opacity': 0.4, visibility: true,
'isBaseLayer': false,'wrapDateLine': true
}
);
map.addLayers([wms,shaded, hybrid, aerial]);
map.setCenter(new OpenLayers.LonLat(-110, 45), 3);
}
</script>
</head>
<body onload="init()">
<h1 id="title">Bing Example</h1>
<div id="tags"></div>
<p id="shortdesc">
Demonstrates the use of Bing layers.
</p>
<div id="map" class="smallmap"></div>
<div id="docs">This example demonstrates the ability to create layers using tiles from Bing maps.</div>
</body>
</html>
4) Openlayers controls
For this example you'll need three files openlayers.js, style.css and theme/style.css. You'll need to create a theme directory for the latter. Note in theme/style.css the icons will need adding to a img directory. I have not done this here, so map icon will not appear.
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>OpenLayers Map Controls Example</title>
<link rel="stylesheet" href="theme/style.css" type="text/css" />
<link rel="stylesheet" href="style.css" type="text/css" />
<script src="OpenLayers.js"></script>
<script type="text/javascript">
var map;
function init(){
map = new OpenLayers.Map('map', {
controls: [
new OpenLayers.Control.Navigation(),
new OpenLayers.Control.PanZoomBar(),
new OpenLayers.Control.LayerSwitcher({'ascending':false}),
new OpenLayers.Control.Permalink(),
new OpenLayers.Control.ScaleLine(),
new OpenLayers.Control.Permalink('permalink'),
new OpenLayers.Control.MousePosition(),
new OpenLayers.Control.OverviewMap(),
new OpenLayers.Control.KeyboardDefaults()
],
numZoomLevels: 6
});
var ol_wms = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://labs.metacarta.com/wms/vmap0",
{layers: 'basic'} );
var jpl_wms = new OpenLayers.Layer.WMS( "NASA Global Mosaic",
"http://t1.hypercube.telascience.org/cgi-bin/landsat7",
{layers: "landsat7"});
var dm_wms = new OpenLayers.Layer.WMS( "DM Solutions Demo",
"http://www2.dmsolutions.ca/cgi-bin/mswms_gmap",
{layers: "bathymetry,land_fn,park,drain_fn,drainage," +
"prov_bound,fedlimit,rail,road,popplace",
transparent: "true", format: "image/png" });
jpl_wms.setVisibility(false);
dm_wms.setVisibility(false);
map.addLayers([ol_wms, jpl_wms, dm_wms]);
if (!map.getCenter()) map.zoomToMaxExtent();
}
</script>
</head>
<body onload="init()">
<h1 id="title">Map Controls Example</h1>
<div id="tags">
</div>
<p id="shortdesc">
Attach zooming, panning, layer switcher, overview map, and permalink map controls to an OpenLayers window.
</p>
<a style="float:right" href="" id="permalink">Permalink</a>
<div id="map" class="smallmap"></div>
<div id="docs"></div>
</body>
</html>
5) Georss
For this example you'll need to download this georss.xml feed (just copy source in your browser)
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>OpenLayers GeoRSS Example</title>
<link rel="stylesheet" href="theme/style.css" type="text/css" />
<link rel="stylesheet" href="style.css" type="text/css" />
<script src="OpenLayers.js"></script>
<script type="text/javascript">
var map, layer;
OpenLayers.ProxyHost = "/proxy/?url=";
function init(){
map = new OpenLayers.Map('map', {maxResolution:'auto'});
layer = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://labs.metacarta.com/wms/vmap0", {layers: 'basic'} );
map.addLayer(layer);
map.setCenter(new OpenLayers.LonLat(0, 0), 0);
map.addControl(new OpenLayers.Control.LayerSwitcher());
}
function addUrl() {
var urlObj = OpenLayers.Util.getElement('url');
var value = urlObj.value;
var parts = value.split("/");
var newl = new OpenLayers.Layer.GeoRSS( parts[parts.length-1], value);
map.addLayer(newl);
urlObj.value = "";
}
</script>
</head>
<body onload="init()">
<h1 id="title">GeoRSS Example</h1>
<div id="tags"></div>
<p id="shortdesc">
Display a couple of locally cached georss feeds on an a basemap.
</p>
<div id="map" class="smallmap"></div>
<div id="docs">
<p>This demo uses the OpenLayers GeoRSS parser, which supports GeoRSS Simple and W3C GeoRSS. Only points are
currently supported. The OpenLayers GeoRSS parser will automatically connect an information bubble to the map
markers, similar to Google maps. In addition, the parser can use custom PNG icons for markers. A sample GeoRSS
file (georss.xml) is included.
<form onsubmit="return false;">
GeoRSS URL: <input type="text" id="url" size="50" value="georss.xml" />
<input type="submit" onclick="addUrl(); return false;" value="Load Feed" onsubmit="addUrl(); return false;" />
</form>
<p>The above input box allows the input of a URL to a GeoRSS feed. This feed can be local to the HTML page --
for example, entering 'georss.xml' will work by default, because there is a local file in the directory called
georss.xml -- or, with a properly set up ProxyHost variable (as is used here), it will be able to load any
HTTP URL which contains GeoRSS and display it. Anything else will simply have no effect.</p>
</div>
</body>
</html>
There are so many examples online. I walked through some, here is a list of just some of the functionality:
Openlayers
Overview map
Random pan
Layers on off tab
Arcgis restful call
Arcims combine
Bing layers
Add box to map
Buffer how many tiles are included outside the view area
Map click events
Draggable rectangle
Fractional zoom (rectangle zoom)
Adding points, lines and polygons
Toolbar - points, lines and polygons
Digitising
Eventing
GeoRSS – Flickr, marker
As a newbie I took out a few code examples:
1) Basic map
<html>
<head>
<title>OpenLayers Example</title>
<script
src="http://openlayers.org/api/OpenLayers.js"></script>
</head>
<body>
<div style="width:100%; height:100%" id="map"></div>
<script defer="defer" type="text/javascript">
var map = new OpenLayers.Map('map');
var wms = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://labs.metacarta.com/wms/vmap0", {layers: 'basic'} );
map.addLayer(wms);
map.zoomToMaxExtent();
</script>
</body>
</html>
2) WMS map
<html>
<head>
<title>OpenLayers Example</title>
<script
src="http://openlayers.org/api/OpenLayers.js"></script>
</head>
<body>
<div style="width:100%; height:100%" id="map"></div>
<script defer="defer" type="text/javascript">
var map = new OpenLayers.Map('map');
var wms = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://labs.metacarta.com/wms/vmap0", {layers: 'basic'} );
map.addLayer(wms);
map.zoomToMaxExtent();
</script>
</body>
</html>
3) Locally running WMS
<html xmlns="http://www.w3.org/1999/xhtml">
<script
src="http://openlayers.org/api/OpenLayers.js"></script>
</head>
<body>
<div style="width:100%; height:100%" id="map"></div>
<script defer="defer" type="text/javascript">
var map = new OpenLayers.Map('map');
var wms = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://localhost:8080/geoserver/wms", {layers: 'topp:states_ugl'} );
map.addLayer(wms);
map.zoomToMaxExtent();
</script>
</html>
4) Local WMS with Bing (note you will need to copy the OpenLayers.js file from
http://openlayers.org/dev/OpenLayers.js)
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>OpenLayers Bing Example</title>
<link rel="stylesheet" href="../theme/default/style.css" type="text/css" />
<link rel="stylesheet" href="style.css" type="text/css" />
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<script src="http://ecn.dev.virtualearth.net/mapcontrol/mapcontrol.ashx?v=6.2&mkt=en-us"></script>
<script src="OpenLayers.js"></script>
<script>
var map;
function init(){
map = new OpenLayers.Map("map");
map.addControl(new OpenLayers.Control.LayerSwitcher());
var shaded = new OpenLayers.Layer.VirtualEarth("Shaded", {
type: VEMapStyle.Shaded
});
var hybrid = new OpenLayers.Layer.VirtualEarth("Hybrid", {
type: VEMapStyle.Hybrid
});
var aerial = new OpenLayers.Layer.VirtualEarth("Aerial", {
type: VEMapStyle.Aerial
});
var wms = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://localhost:8080/geoserver/wms", {layers: 'topp:states_ugl'},
{
'opacity': 0.4, visibility: true,
'isBaseLayer': false,'wrapDateLine': true
}
);
map.addLayers([wms,shaded, hybrid, aerial]);
map.setCenter(new OpenLayers.LonLat(-110, 45), 3);
}
</script>
</head>
<body onload="init()">
<h1 id="title">Bing Example</h1>
<div id="tags"></div>
<p id="shortdesc">
Demonstrates the use of Bing layers.
</p>
<div id="map" class="smallmap"></div>
<div id="docs">This example demonstrates the ability to create layers using tiles from Bing maps.</div>
</body>
</html>
4) Openlayers controls
For this example you'll need three files openlayers.js, style.css and theme/style.css. You'll need to create a theme directory for the latter. Note in theme/style.css the icons will need adding to a img directory. I have not done this here, so map icon will not appear.
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>OpenLayers Map Controls Example</title>
<link rel="stylesheet" href="theme/style.css" type="text/css" />
<link rel="stylesheet" href="style.css" type="text/css" />
<script src="OpenLayers.js"></script>
<script type="text/javascript">
var map;
function init(){
map = new OpenLayers.Map('map', {
controls: [
new OpenLayers.Control.Navigation(),
new OpenLayers.Control.PanZoomBar(),
new OpenLayers.Control.LayerSwitcher({'ascending':false}),
new OpenLayers.Control.Permalink(),
new OpenLayers.Control.ScaleLine(),
new OpenLayers.Control.Permalink('permalink'),
new OpenLayers.Control.MousePosition(),
new OpenLayers.Control.OverviewMap(),
new OpenLayers.Control.KeyboardDefaults()
],
numZoomLevels: 6
});
var ol_wms = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://labs.metacarta.com/wms/vmap0",
{layers: 'basic'} );
var jpl_wms = new OpenLayers.Layer.WMS( "NASA Global Mosaic",
"http://t1.hypercube.telascience.org/cgi-bin/landsat7",
{layers: "landsat7"});
var dm_wms = new OpenLayers.Layer.WMS( "DM Solutions Demo",
"http://www2.dmsolutions.ca/cgi-bin/mswms_gmap",
{layers: "bathymetry,land_fn,park,drain_fn,drainage," +
"prov_bound,fedlimit,rail,road,popplace",
transparent: "true", format: "image/png" });
jpl_wms.setVisibility(false);
dm_wms.setVisibility(false);
map.addLayers([ol_wms, jpl_wms, dm_wms]);
if (!map.getCenter()) map.zoomToMaxExtent();
}
</script>
</head>
<body onload="init()">
<h1 id="title">Map Controls Example</h1>
<div id="tags">
</div>
<p id="shortdesc">
Attach zooming, panning, layer switcher, overview map, and permalink map controls to an OpenLayers window.
</p>
<a style="float:right" href="" id="permalink">Permalink</a>
<div id="map" class="smallmap"></div>
<div id="docs"></div>
</body>
</html>
5) Georss
For this example you'll need to download this georss.xml feed (just copy source in your browser)
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>OpenLayers GeoRSS Example</title>
<link rel="stylesheet" href="theme/style.css" type="text/css" />
<link rel="stylesheet" href="style.css" type="text/css" />
<script src="OpenLayers.js"></script>
<script type="text/javascript">
var map, layer;
OpenLayers.ProxyHost = "/proxy/?url=";
function init(){
map = new OpenLayers.Map('map', {maxResolution:'auto'});
layer = new OpenLayers.Layer.WMS( "OpenLayers WMS",
"http://labs.metacarta.com/wms/vmap0", {layers: 'basic'} );
map.addLayer(layer);
map.setCenter(new OpenLayers.LonLat(0, 0), 0);
map.addControl(new OpenLayers.Control.LayerSwitcher());
}
function addUrl() {
var urlObj = OpenLayers.Util.getElement('url');
var value = urlObj.value;
var parts = value.split("/");
var newl = new OpenLayers.Layer.GeoRSS( parts[parts.length-1], value);
map.addLayer(newl);
urlObj.value = "";
}
</script>
</head>
<body onload="init()">
<h1 id="title">GeoRSS Example</h1>
<div id="tags"></div>
<p id="shortdesc">
Display a couple of locally cached georss feeds on an a basemap.
</p>
<div id="map" class="smallmap"></div>
<div id="docs">
<p>This demo uses the OpenLayers GeoRSS parser, which supports GeoRSS Simple and W3C GeoRSS. Only points are
currently supported. The OpenLayers GeoRSS parser will automatically connect an information bubble to the map
markers, similar to Google maps. In addition, the parser can use custom PNG icons for markers. A sample GeoRSS
file (georss.xml) is included.
<form onsubmit="return false;">
GeoRSS URL: <input type="text" id="url" size="50" value="georss.xml" />
<input type="submit" onclick="addUrl(); return false;" value="Load Feed" onsubmit="addUrl(); return false;" />
</form>
<p>The above input box allows the input of a URL to a GeoRSS feed. This feed can be local to the HTML page --
for example, entering 'georss.xml' will work by default, because there is a local file in the directory called
georss.xml -- or, with a properly set up ProxyHost variable (as is used here), it will be able to load any
HTTP URL which contains GeoRSS and display it. Anything else will simply have no effect.</p>
</div>
</body>
</html>
There are so many examples online. I walked through some, here is a list of just some of the functionality:
Openlayers
Overview map
Random pan
Layers on off tab
Arcgis restful call
Arcims combine
Bing layers
Add box to map
Buffer how many tiles are included outside the view area
Map click events
Draggable rectangle
Fractional zoom (rectangle zoom)
Adding points, lines and polygons
Toolbar - points, lines and polygons
Digitising
Eventing
GeoRSS – Flickr, marker
Wednesday, September 16, 2009
TileCache and Google Maps
Found the following excellent entry from Christopher Schmidt blog. I'll give it a whirl and post my results.
MapServer and TileCache
Spent some time today looking at using TileCache with MapServer. I used in part these docs. Other useful docs are here from Metacarta. I walked through the following (note I am using my work with Mapserver described in an earlier post):
1) Installed Python
2) Downloaded TileCache
3) Unzipped the TileCache directory, ran the setup executable and copied the contents under the directory into C:\ms4w\Apache\cgi-bin
4) Opened C:\ms4w\Apache\cgi-bin\tilecache.cgi and changed the top line to:
#!C:/Python25/python.exe -u
5) I added the following to the C:\ms4w\Apache\cgi-bin\tilecache.cfg file
[mapserver]
type=WMS
url=http://localhost:81/cgi-bin/mapserv.exe?map=/ms4w/Apache/htdocs/wms_mapserver/data/ms_wms.map
extension=png
layers=states_ugl
SRS=EPSG:4326
bbox=-97.6169,39.9179,-81.745,51.0875
6) Running the following:
http://localhost:81/cgi-bin/tilecache.cgi?layers=mapserver&service=WMS&version=1.1.1&request=GetMap&srs=EPSG:4326&bbox=-97.6169,39.9179,-81.745,51.0875&format=image/png&width=400&height=300
gives the map image served by MapServer.
1) Installed Python
2) Downloaded TileCache
3) Unzipped the TileCache directory, ran the setup executable and copied the contents under the directory into C:\ms4w\Apache\cgi-bin
4) Opened C:\ms4w\Apache\cgi-bin\tilecache.cgi and changed the top line to:
#!C:/Python25/python.exe -u
5) I added the following to the C:\ms4w\Apache\cgi-bin\tilecache.cfg file
[mapserver]
type=WMS
url=http://localhost:81/cgi-bin/mapserv.exe?map=/ms4w/Apache/htdocs/wms_mapserver/data/ms_wms.map
extension=png
layers=states_ugl
SRS=EPSG:4326
bbox=-97.6169,39.9179,-81.745,51.0875
6) Running the following:
http://localhost:81/cgi-bin/tilecache.cgi?layers=mapserver&service=WMS&version=1.1.1&request=GetMap&srs=EPSG:4326&bbox=-97.6169,39.9179,-81.745,51.0875&format=image/png&width=400&height=300
gives the map image served by MapServer.
MapQuest Flex API Samples
Now leaning towards the Mapquest Flex api. I spied the following demo applications. This demonstrates with source code, some of the core functionality.
On a side note. The AIR application Tour de Flex is well worth installing if you build Flex apps. From a mapping perspective they demo a number of the main mapping API's.
On a side note. The AIR application Tour de Flex is well worth installing if you build Flex apps. From a mapping perspective they demo a number of the main mapping API's.
Tuesday, September 15, 2009
Flex Developer List
Sometimes useful people to ask for solutions for those hard to solve problems. Here is one persons experts list.
Mapping API's
I'll admit to being somewhat locked into the Modest Maps Flex API. As recent blog entries suggest, I've started looking at combining WMS and WFS data - served from MapeServer or Geoserver - with basemaps. I've had a number of people approach me about this combination, plus I've wanted the excuse the exlpore further.
Another area which I have started investigating are other Flex/Flash mapping API's. The main four are ESRI ArcMap, Google maps, Yahoo and Mapquest. I came across the following article written by Kevin Hoyt of Adobe. He compared the Google, Yahoo and Mapquest last year. Finding Mapquest the richest and best supported. He did a presentation which included Modest maps and ESRI's API. Its worth a read.
For me, after chatting with Mapquest at Where 2.0 this year, and based on what I have read, I'll turn my attention here first.
Another area which I have started investigating are other Flex/Flash mapping API's. The main four are ESRI ArcMap, Google maps, Yahoo and Mapquest. I came across the following article written by Kevin Hoyt of Adobe. He compared the Google, Yahoo and Mapquest last year. Finding Mapquest the richest and best supported. He did a presentation which included Modest maps and ESRI's API. Its worth a read.
For me, after chatting with Mapquest at Where 2.0 this year, and based on what I have read, I'll turn my attention here first.
Sunday, September 13, 2009
Modest Maps, WMS and Overview
Looking at the docs on wms and multiple providers the following proved a useful link:
http://getsatisfaction.com/modestmaps/topics/how_to_layer_two_maps_with_different_providers_for_blending_alpha_transparency_etc
I'll post my code in due course.
On a related note, here is a URL for an overview map with Modest maps
http://blog.modestmaps.com/2008/11/hello-world/
http://getsatisfaction.com/modestmaps/topics/how_to_layer_two_maps_with_different_providers_for_blending_alpha_transparency_etc
I'll post my code in due course.
On a related note, here is a URL for an overview map with Modest maps
http://blog.modestmaps.com/2008/11/hello-world/
GeoServer and WMS
Geoserver is a truly fabulous open source geospatial server. Much easier to set and configure than Mapserver. The documentation provided is extremely comprehensive. See:
http://docs.geoserver.org/1.7.6/user/getting-started/web-admin-quickstart/index.html
Here are the notes I made as I walked through setting things up.
a) Setting up geoserver
I am using the portable gis control panel (see earlier blog entry). Once installed it sits directly under c:/.When you open it you have the option (under web modules) to start Geoserver.
Access Geoserver via:
http://localhost:8080/geoserver
admin/password are the default credentials. If you need to change them look at:
C:\Program Files\GeoServer 1.7.6\data_dir\security\user.properties
b) Adding shapefile
Under the data directory for geoserver add some new data in the data directory:
C:\Program Files\GeoServer 1.7.6\data_dir\data\
In the web interface click on data -> datastores -> new. Add name and type. Next screen add path to data and data name eg. nyc_roads.shp. Now click submit. The next screen should be the featuretype editor. Now from drop down select the type eg. line add the srs, then click generate. Now click submit and finally apply. Note these two steps in this order are critical in the process.
In the web interface under data->datastores you should now see your new data
You can view the data in welcome->demo->map preview
c) WMS
In config screen choose wms. Next choose contents and add the layer you just created in this screen. You can get the SRS info from the featuretype editor under data
One of the amazingly cool things about geoserver is the demos page in the web interface. From here you can view the results of various requests. Choose sample requests to see these options; wms, wfs etc. Also from here you can select map preview. From here you can see your newly loaded data. Another terrific thing about geoserver is the integration of openlayers. Data can be viewed directly in the geoserver interface with openlayers
d) Geowebcache
Another terrific part of geoserver is the integration of geowebcache. There is a nice guide to this here:
http://geoserver.org/display/GEOSDOC/GeoServer+GeoWebCache+Configuration
Key directory is C:\Program Files\GeoServer 1.7.6\data_dir\gwc. Go to:
http://localhost:8080/geoserver/gwc/demo
Here you can see all of your layers and ‘seed this layer’. Seeding is generating tiles. When you select seed this layer you will see under the gwc directory your newly generated tiles.
If you go to the demo page and view your data. In openlayers you have the option to view the data as a single image or tiled. The latter will show your newly generated tiles.
If you wish to see the data in google earth. Open Google earth, choose add->network link. Add a name and the url below to the pop up.
http://localhost:8080/geoserver/gwc/service/kml/topp:nyc_roads.png.kml
By selecting the named link in google earth (under my places) you should see your data.
http://docs.geoserver.org/1.7.6/user/getting-started/web-admin-quickstart/index.html
Here are the notes I made as I walked through setting things up.
a) Setting up geoserver
I am using the portable gis control panel (see earlier blog entry). Once installed it sits directly under c:/.When you open it you have the option (under web modules) to start Geoserver.
Access Geoserver via:
http://localhost:8080/geoserver
admin/password are the default credentials. If you need to change them look at:
C:\Program Files\GeoServer 1.7.6\data_dir\security\user.properties
b) Adding shapefile
Under the data directory for geoserver add some new data in the data directory:
C:\Program Files\GeoServer 1.7.6\data_dir\data\
In the web interface click on data -> datastores -> new. Add name and type. Next screen add path to data and data name eg. nyc_roads.shp. Now click submit. The next screen should be the featuretype editor. Now from drop down select the type eg. line add the srs, then click generate. Now click submit and finally apply. Note these two steps in this order are critical in the process.
In the web interface under data->datastores you should now see your new data
You can view the data in welcome->demo->map preview
c) WMS
In config screen choose wms. Next choose contents and add the layer you just created in this screen. You can get the SRS info from the featuretype editor under data
One of the amazingly cool things about geoserver is the demos page in the web interface. From here you can view the results of various requests. Choose sample requests to see these options; wms, wfs etc. Also from here you can select map preview. From here you can see your newly loaded data. Another terrific thing about geoserver is the integration of openlayers. Data can be viewed directly in the geoserver interface with openlayers
d) Geowebcache
Another terrific part of geoserver is the integration of geowebcache. There is a nice guide to this here:
http://geoserver.org/display/GEOSDOC/GeoServer+GeoWebCache+Configuration
Key directory is C:\Program Files\GeoServer 1.7.6\data_dir\gwc. Go to:
http://localhost:8080/geoserver/gwc/demo
Here you can see all of your layers and ‘seed this layer’. Seeding is generating tiles. When you select seed this layer you will see under the gwc directory your newly generated tiles.
If you go to the demo page and view your data. In openlayers you have the option to view the data as a single image or tiled. The latter will show your newly generated tiles.
If you wish to see the data in google earth. Open Google earth, choose add->network link. Add a name and the url below to the pop up.
http://localhost:8080/geoserver/gwc/service/kml/topp:nyc_roads.png.kml
By selecting the named link in google earth (under my places) you should see your data.
Friday, September 11, 2009
WMS and MapServer
I'm in the process of setting up Mapserver to serve up maps via WMS. It has been a while. The steps I went through are as follows:
1) Download MS4W . This is a package which bundles Mapserver with PHP and Apache. Saves time on configuration. I set the Apache port to 81, since I already have another version running on 80. The default location of all installed files is c:/ms4w.
2) Installed Quantum GIS. Again you can use another bundled package which includes geoserver and many other open source tools. Alternatively you can get the software direct.
3) Quantum GIS contains a nifty plug in for generating .map or mapfiles for Mapserver (the alternative is to write them by hand which can be a major pain). This can be done via the plugin menu option. Once installed, this is the plug in window:
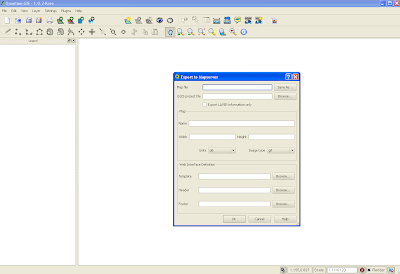
Two things to remember. First, create an empty .map file in your favourite text editor. In the plug in interface, you need to point to an existing .map file. The Quantum plug in will overwrite this file. Second, I found it easier to put the .map file and shapefiles (at least for this initial trial) in the same directory.
You should now have a working mapfile. Now for WMS. You will need to edit the generated mapfile to add additional parameters. This and the required url parameters are a pain. After much searching I found these two links very useful:
http://www.mapserver.org/ogc/wms_server.html#table-of-contents
http://ms.gis.umn.edu:8081/ms_plone/docs/howto/mapcontext
I'll not walk too much through the additions, but they mostly involve adding elements under the METADATA tag. Here is my working mapfile:
# Map file created from QGIS project file C:/matts/generatefile/test.qgs
# Edit this file to customize for your map interface
# (Created with PyQgis MapServer Export plugin)
MAP
NAME test
# Map image size
SIZE 400 300
#UNITS meters
EXTENT -97.616877 39.917875 -81.745000 51.087523
PROJECTION
'proj=longlat'
'ellps=WGS84'
'datum=WGS84'
'no_defs'
''
END
# Background color for the map canvas -- change as desired
# IMAGECOLOR 192 192 192
#IMAGEQUALITY 95
IMAGETYPE png
OUTPUTFORMAT
NAME png
DRIVER "GD/PNG"
MIMETYPE "image/png"
IMAGEMODE RGB
EXTENSION "png"
FORMATOPTION "INTERLACE=OFF"
END
# Legend
LEGEND
IMAGECOLOR 255 255 255
STATUS ON
KEYSIZE 18 12
LABEL
TYPE BITMAP
SIZE MEDIUM
COLOR 0 0 89
END
END
# Web interface definition. Only the template parameter
# is required to display a map. See MapServer documentation
WEB
# Set IMAGEPATH to the path where MapServer should
# write its output.
IMAGEPATH '/tmp/'
# Set IMAGEURL to the url that points to IMAGEPATH
# as defined in your web server configuration
IMAGEURL 'http://localhost:81/wms_mapserver/tmp'
# WMS server settings
METADATA
'wms_title' 'test'
'wms_onlineresource' 'http://localhost:81/cgi-bin/mapserv.exe?map=C:/ms4w/Apache/htdocs/wms_mapserver/data/ms_wms.map&'
'wms_srs' 'EPSG:4326'
"wms_extent" "201621.496941 -294488.285333 1425518.020722 498254.511514"
#"wfs_title" "test WFS Demo Server" ## REQUIRED
END
#Scale range at which web interface will operate
# Template and header/footer settings
# Only the template parameter is required to display a map. See MapServer documentation
END
LAYER
NAME 'states_ugl'
TYPE POLYGON
DATA 'C:/ms4w/Apache/htdocs/wms_mapserver/data/states_ugl.shp'
METADATA
"wms_name" "states"
"wms_server_version" "1.1.1"
"wms_format" "image/png"
"wms_srs" "EPSG:4326"
"wms_title" "States" ##required
"wms_extent" "201621.496941 -294488.285333 1425518.020722 498254.511514"
"wms_onlineresource" "http://localhost:81/cgi-bin/mapserv.exe?map=C:/ms4w/Apache/htdocs/wms_mapserver/data/ms_wms.map?"
#"wfs_version" "1.1.0"
# "wfs_srs" "EPSG:4326"
# "wfs_typename" "statefs"
# "wfs_request_method" "GET"
# "wfs_service" "WFS"
#"wfs_title" "statefs" ## REQUIRED
#"gml_featureid" "ID" ## REQUIRED
#"gml_include_items" "all" ## Optional (serves all attributes for layer)
END
#CONNECTIONTYPE WMS
STATUS ON
TRANSPARENCY 100
PROJECTION
'proj=longlat'
'ellps=WGS84'
'datum=WGS84'
'no_defs'
''
END
CLASS
NAME 'states_ugl'
STYLE
SYMBOL 0
SIZE 2
OUTLINECOLOR 0 0 0
COLOR 144 50 207
END
END
END
END
As you can see this sits in the following directory with my shapefile:
C:/ms4w/Apache/htdocs/wms_mapserver/data
I did my testing using Internet Explorer. As mentioned, the required url parameters are a pain. Here are the two key calls; getCapabilities:
http://localhost:81/cgi-bin/mapserv.exe?map=/ms4w/Apache/htdocs/wms_mapserver/data/ms_wms.map&SERVICE=WMS&VERSION=1.1.1&REQUEST=GetCapabilities
and getMap. Note all parameters except styles are required:
http://localhost:81/cgi-bin/mapserv.exe?map=/ms4w/Apache/htdocs/wms_mapserver/data/ms_wms.map&service=WMS&version=1.1.1&request=GetMap&layers=states_ugl&srs=EPSG:4326&bbox=-97.6169,39.9179,-81.745,51.0875&format=image/png&width=400&height=300&styles=default
I struggled for a while on the getMap call. One problem I realised was the BBox parameters you need to get from getCapabilities (I grabbed it directly from the mapfile). I also initially added connectiontype "wms" for the layer in the mapfile which broke everything - actually i could not generate an image. As soon as that was removed bingo.
Grab this source, unrar and place in your C:/ms4w/Apache/htdocs. You should now be able to run the above.
1) Download MS4W . This is a package which bundles Mapserver with PHP and Apache. Saves time on configuration. I set the Apache port to 81, since I already have another version running on 80. The default location of all installed files is c:/ms4w.
2) Installed Quantum GIS. Again you can use another bundled package which includes geoserver and many other open source tools. Alternatively you can get the software direct.
3) Quantum GIS contains a nifty plug in for generating .map or mapfiles for Mapserver (the alternative is to write them by hand which can be a major pain). This can be done via the plugin menu option. Once installed, this is the plug in window:
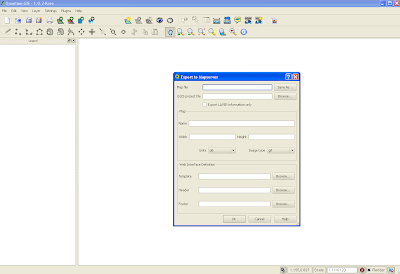
Two things to remember. First, create an empty .map file in your favourite text editor. In the plug in interface, you need to point to an existing .map file. The Quantum plug in will overwrite this file. Second, I found it easier to put the .map file and shapefiles (at least for this initial trial) in the same directory.
You should now have a working mapfile. Now for WMS. You will need to edit the generated mapfile to add additional parameters. This and the required url parameters are a pain. After much searching I found these two links very useful:
http://www.mapserver.org/ogc/wms_server.html#table-of-contents
http://ms.gis.umn.edu:8081/ms_plone/docs/howto/mapcontext
I'll not walk too much through the additions, but they mostly involve adding elements under the METADATA tag. Here is my working mapfile:
# Map file created from QGIS project file C:/matts/generatefile/test.qgs
# Edit this file to customize for your map interface
# (Created with PyQgis MapServer Export plugin)
MAP
NAME test
# Map image size
SIZE 400 300
#UNITS meters
EXTENT -97.616877 39.917875 -81.745000 51.087523
PROJECTION
'proj=longlat'
'ellps=WGS84'
'datum=WGS84'
'no_defs'
''
END
# Background color for the map canvas -- change as desired
# IMAGECOLOR 192 192 192
#IMAGEQUALITY 95
IMAGETYPE png
OUTPUTFORMAT
NAME png
DRIVER "GD/PNG"
MIMETYPE "image/png"
IMAGEMODE RGB
EXTENSION "png"
FORMATOPTION "INTERLACE=OFF"
END
# Legend
LEGEND
IMAGECOLOR 255 255 255
STATUS ON
KEYSIZE 18 12
LABEL
TYPE BITMAP
SIZE MEDIUM
COLOR 0 0 89
END
END
# Web interface definition. Only the template parameter
# is required to display a map. See MapServer documentation
WEB
# Set IMAGEPATH to the path where MapServer should
# write its output.
IMAGEPATH '/tmp/'
# Set IMAGEURL to the url that points to IMAGEPATH
# as defined in your web server configuration
IMAGEURL 'http://localhost:81/wms_mapserver/tmp'
# WMS server settings
METADATA
'wms_title' 'test'
'wms_onlineresource' 'http://localhost:81/cgi-bin/mapserv.exe?map=C:/ms4w/Apache/htdocs/wms_mapserver/data/ms_wms.map&'
'wms_srs' 'EPSG:4326'
"wms_extent" "201621.496941 -294488.285333 1425518.020722 498254.511514"
#"wfs_title" "test WFS Demo Server" ## REQUIRED
END
#Scale range at which web interface will operate
# Template and header/footer settings
# Only the template parameter is required to display a map. See MapServer documentation
END
LAYER
NAME 'states_ugl'
TYPE POLYGON
DATA 'C:/ms4w/Apache/htdocs/wms_mapserver/data/states_ugl.shp'
METADATA
"wms_name" "states"
"wms_server_version" "1.1.1"
"wms_format" "image/png"
"wms_srs" "EPSG:4326"
"wms_title" "States" ##required
"wms_extent" "201621.496941 -294488.285333 1425518.020722 498254.511514"
"wms_onlineresource" "http://localhost:81/cgi-bin/mapserv.exe?map=C:/ms4w/Apache/htdocs/wms_mapserver/data/ms_wms.map?"
#"wfs_version" "1.1.0"
# "wfs_srs" "EPSG:4326"
# "wfs_typename" "statefs"
# "wfs_request_method" "GET"
# "wfs_service" "WFS"
#"wfs_title" "statefs" ## REQUIRED
#"gml_featureid" "ID" ## REQUIRED
#"gml_include_items" "all" ## Optional (serves all attributes for layer)
END
#CONNECTIONTYPE WMS
STATUS ON
TRANSPARENCY 100
PROJECTION
'proj=longlat'
'ellps=WGS84'
'datum=WGS84'
'no_defs'
''
END
CLASS
NAME 'states_ugl'
STYLE
SYMBOL 0
SIZE 2
OUTLINECOLOR 0 0 0
COLOR 144 50 207
END
END
END
END
As you can see this sits in the following directory with my shapefile:
C:/ms4w/Apache/htdocs/wms_mapserver/data
I did my testing using Internet Explorer. As mentioned, the required url parameters are a pain. Here are the two key calls; getCapabilities:
http://localhost:81/cgi-bin/mapserv.exe?map=/ms4w/Apache/htdocs/wms_mapserver/data/ms_wms.map&SERVICE=WMS&VERSION=1.1.1&REQUEST=GetCapabilities
and getMap. Note all parameters except styles are required:
http://localhost:81/cgi-bin/mapserv.exe?map=/ms4w/Apache/htdocs/wms_mapserver/data/ms_wms.map&service=WMS&version=1.1.1&request=GetMap&layers=states_ugl&srs=EPSG:4326&bbox=-97.6169,39.9179,-81.745,51.0875&format=image/png&width=400&height=300&styles=default
I struggled for a while on the getMap call. One problem I realised was the BBox parameters you need to get from getCapabilities (I grabbed it directly from the mapfile). I also initially added connectiontype "wms" for the layer in the mapfile which broke everything - actually i could not generate an image. As soon as that was removed bingo.
Grab this source, unrar and place in your C:/ms4w/Apache/htdocs. You should now be able to run the above.
Wednesday, August 26, 2009
Flash Flex Components
The integration of Flash and Flex remains somewhat challenging. They both continue to live in separate worlds (Catalyst seems as though it is a step towards bridging this gap). The integration is not seemless. One way I have mentioned before is to load a Flash swf in Flex then using eventing to communicate back and forth. I just came across the following video which explains how to convert a Flash application to a Flex component. It proved a little tricky at first, but when you have it working it is an excellent solution. The main steps are:
1) Download the Flex Component kit from Adobe.
2) Inside of Flash the applications class should be written:
package {
import mx.flash.UIMovieClip;
public dynamic class lessonNavigation extends UIMovieClip
{
}
}
3) In the Flash IDE menu select Commands -> Convert Symbol to Flex component. This generates a SWC
4) Copy this to your Flex project and add the swc to the projects library path.
5) You should now be able to access the component from Flex.
1) Download the Flex Component kit from Adobe.
2) Inside of Flash the applications class should be written:
package {
import mx.flash.UIMovieClip;
public dynamic class lessonNavigation extends UIMovieClip
{
}
}
3) In the Flash IDE menu select Commands -> Convert Symbol to Flex component. This generates a SWC
4) Copy this to your Flex project and add the swc to the projects library path.
5) You should now be able to access the component from Flex.
Friday, August 7, 2009
OpenLayers
OpenLayers is a great open source mapping client. I've not worked with it directly, since I'm focused on building mapping RIA's using Flex and Flash. But I've seen some terrific sites built using OpenLayers. Cycling Switzerland is such an example. I really like the design. Only downside is if you uncheck a checkbox within a group eg. hiking, all routes reload as against turning off just this subset
Wednesday, August 5, 2009
Building RIA maps
It took a while but my article for Adobe on building RIA maps in Flex has been published.
Thursday, July 30, 2009
Loading Modules in Flex
I have in the works an article on map interfaces. Its challenging to provide the map and metadata on, say, a point clicked on the map. Maybe its a marker for a house for sale. I click on the marker, where do I display the price, images, descriptions etc? Pop up, draggable boxes, open a new page. Lots of choices, some better than others. Flex actually increases the possible cool options here.
My article was driven by a new local search application I am writing. I'm debating whether to load modules within the application. With this in mind, I have built a demo. Right click and you can view the code. This does the following:
1) Loads modules on the fly based on user button click; next or previous(note the use of an interface)
2) Allows the main application to call methods in the loaded module.
3) The modules listen to events fired from the main application
4) The text in the main application is localised; so button labels can be switched from English to Spanish.
Take a look at the code or download it to test. In the project properties ->flex modules, you will need to have the two modules included. So they are compiled with the application.
The demo is potentially a base for building mapping applications. Or applications which include a mapping module.
My article was driven by a new local search application I am writing. I'm debating whether to load modules within the application. With this in mind, I have built a demo. Right click and you can view the code. This does the following:
1) Loads modules on the fly based on user button click; next or previous(note the use of an interface)
2) Allows the main application to call methods in the loaded module.
3) The modules listen to events fired from the main application
4) The text in the main application is localised; so button labels can be switched from English to Spanish.
Take a look at the code or download it to test. In the project properties ->flex modules, you will need to have the two modules included. So they are compiled with the application.
The demo is potentially a base for building mapping applications. Or applications which include a mapping module.
Labels:
consumer maps,
events,
Flex modules,
localization,
map interfaces
John Peel and Redwoods
Just back from vacation in Bay area, California. Those redwood forests are wonderlands to me. To think the place was once filled with such trees. How could we let loggers cut them all down .... I digress.
Plenty to write about on the conference scene. With vacations and work I have had to follow The ESRI business and GeoWeb conferences via twitter and blogs. Still I'll gather peoples feedback in my next post and add some comment.
With vacation thoughts still in mind. I'm a big music fan. Mostly my interest is in new bands, from all genres. One of my heroes (if I can use such a nonsense term) was John Peel. A unique radio DJ and person. His shows were broadcast on BBC radio 1 from his home near Ipswich in England. Often he would begin his introduction to "the new song by Extreme Noise Terror", by describing the antics of the wasps outside his window. His two hour show was one of life's pleasures. Like many I miss his wit and observations (he passed away while on vacation in Peru in 2004). Anyway, a book of his music reviews was released at the end of last year. There is one review of the book on Amazon by Richard Fudge, I felt it needed reprinting. Not only for how beautifully it is written but its content:
As far as I can tell, John Peel was a fine human being and as avid and influential a music fan as ever existed. This collection of columns published in various British magazines from the 1970s till his death in 2004 shows what an amazingly good writer he was too. I haven't enjoyed a music book this much in I don't know how long--chiefly because his wry sense of humor and nimble wordcraft are hilarious. As I read the book, I would share occasional columns with my wife--and there were times when we laughed so hard we gasped for breath.
As a DJ, Peel was eager to share any music that he found interesting or exciting, and as a result listeners to his radio show could potentially hear anything from anywhere by anybody. There are elements of the unusual in the book, but for the most part Peel deals with mainstream music and culture (certainly mainstream if you're an indie or World music fan) and this book works somewhat as a one-man overview of music from the last half of the 20th century. Having said that, Peel is indelibly British and his columns are therefore packed with references to British life and culture; as an ex-pat I enjoyed this greatly, but someone not so acquainted with UK life will certainly feel left out at times. Don't let this scare you away. Peel's reflections on the musicians who dazzled him (and his derision of the performers and music-industry types who had little going on but a well-marketed pose) are fascinating and insightful, and they resonate with the enthusiasm that was so much of Peel's radio demeanor.
Music writing can too often be pompously self-important. Peel, always self-effacing, is serious, intelligent, articulate, analytical, informed, and so on--but he steers well clear of the pompous.
What is also gratifying about Peel's outlook on life is his appreciation of the decency of human kindness, and his frequently practiced understanding that a few beers can greatly enhance the human experience. I imagine he would have been a great guy to hang out with--and reading this book was a good deal like hanging out with a great guy.
Plenty to write about on the conference scene. With vacations and work I have had to follow The ESRI business and GeoWeb conferences via twitter and blogs. Still I'll gather peoples feedback in my next post and add some comment.
With vacation thoughts still in mind. I'm a big music fan. Mostly my interest is in new bands, from all genres. One of my heroes (if I can use such a nonsense term) was John Peel. A unique radio DJ and person. His shows were broadcast on BBC radio 1 from his home near Ipswich in England. Often he would begin his introduction to "the new song by Extreme Noise Terror", by describing the antics of the wasps outside his window. His two hour show was one of life's pleasures. Like many I miss his wit and observations (he passed away while on vacation in Peru in 2004). Anyway, a book of his music reviews was released at the end of last year. There is one review of the book on Amazon by Richard Fudge, I felt it needed reprinting. Not only for how beautifully it is written but its content:
As far as I can tell, John Peel was a fine human being and as avid and influential a music fan as ever existed. This collection of columns published in various British magazines from the 1970s till his death in 2004 shows what an amazingly good writer he was too. I haven't enjoyed a music book this much in I don't know how long--chiefly because his wry sense of humor and nimble wordcraft are hilarious. As I read the book, I would share occasional columns with my wife--and there were times when we laughed so hard we gasped for breath.
As a DJ, Peel was eager to share any music that he found interesting or exciting, and as a result listeners to his radio show could potentially hear anything from anywhere by anybody. There are elements of the unusual in the book, but for the most part Peel deals with mainstream music and culture (certainly mainstream if you're an indie or World music fan) and this book works somewhat as a one-man overview of music from the last half of the 20th century. Having said that, Peel is indelibly British and his columns are therefore packed with references to British life and culture; as an ex-pat I enjoyed this greatly, but someone not so acquainted with UK life will certainly feel left out at times. Don't let this scare you away. Peel's reflections on the musicians who dazzled him (and his derision of the performers and music-industry types who had little going on but a well-marketed pose) are fascinating and insightful, and they resonate with the enthusiasm that was so much of Peel's radio demeanor.
Music writing can too often be pompously self-important. Peel, always self-effacing, is serious, intelligent, articulate, analytical, informed, and so on--but he steers well clear of the pompous.
What is also gratifying about Peel's outlook on life is his appreciation of the decency of human kindness, and his frequently practiced understanding that a few beers can greatly enhance the human experience. I imagine he would have been a great guy to hang out with--and reading this book was a good deal like hanging out with a great guy.
Monday, July 20, 2009
Flex Flash Maps
I mentioned in an earlier post my Flash revisit. The Flash map applications in the London Guardian have always impressed me. I thought I could use Flex as the framework and eventing manager and build a series of flash maps. So a Flex Flash combo. Modularity was also something I wanted to explore. The large Flash applications built by some of the flash mapping companies seem unnecessary. Anyway, the application in its base form is available here. Right click to view the code and/or download.

Note: In the right pane you can zoom in and out by selecting a tool and clicking. Alternatively you can simply use the mouse wheel.

Note: In the right pane you can zoom in and out by selecting a tool and clicking. Alternatively you can simply use the mouse wheel.
Simple MVC
I have just posted the code for a very simple MVC. Right click and you can see the code and download it. Its very simple. This should give you a feel for the guts of the MVC architecture. Should make understanding Cairngorm and the rest a little easier.
Thursday, July 16, 2009
Location Intelligence
Introduction
We are in the midst of a geospatial revolution. Geo what I hear you saying? Is this geography; maybe maps? Both and more. Specifically we are talking about location. Location in space; how it is visualized and analyzed. In this article we will introduce the new landscape that is geospatial. We will see how we got to today, define terms, compare platforms and segments. Ultimately, we will help you better understand location and its importance to your business.
Let's start at the Beginning
So what is location? At its most basic we are talking about a point in space; you, me, a house, a store, a sale, maybe a delivery. All entities and events have a location. Every business operates in this space.
So we are talking maps then. Yes and no. Maps are simply a way to visualize space. They have context. So driving maps, show roads and settlements. On thematic maps voting patterns across the US may be represented. Changes in city boundaries over time can be shown on temporal maps. More accurately, we are talking about elements or activities which exist or take place respectively in space. A map is merely the representation of these elements or activities, together or in isolation, in space. Maps are images. Humans are wired to best understand information when it is presented in images. Thus maps are a wonderful way to present complex information in understandable ways.
What is Geospatial
Geo is a prefix for any word dealing in some way with the earth. Spatial is from the word space or the boundless, three-dimensional extent in which objects and events occur with relative position and direction. Geospatial is the visualization and analysis of terrestrial geographic datasets. If “geo” is so important to understanding physical assets and the location of people - two critical components to the commerce of goods and services, and therefore business decisions - why has location been an untapped dimension of business? Why has GIS been in the closet for 25 years? And, so why suddenly has its profile been raised? A brief history might be in order.
Proprietary Software v. Spatial Thinking
Geospatial has been dominated by geographic information systems (GIS) for 25 years. GIS was a specialized niche. Desktop applications like ESRI’s ARC/INFO and ArcView allowed users to display points, lines and polygons stored in spatially enabled databases as maps, and to perform spatial analysis on this data. Thus if a store wished to build a new branch it could use a GIS to analyze proposed areas in terms of maybe demographics; we want a high percentage of families with a certain household income within a 5 mile radius of our new store.
The Role of Money
In 1996 Mapquest became the first mapping Internet site, enabling users to turn to the Web for driving directions. ESRI released its IMS product line; MapObjects IMS and ArcView IMS, in 1997. Giving developers tools to build online GIS applications. Though these were key developments it was not until 2005 with the launch of Google Maps that the geospatial revolution truly began. So why was Googles entry into geospatial so impactful? For a number of reasons. First was the amount of money put behind the launch. Take hardware for example, it is thought Google now stores many thousands of terrabytes of spatial data. At the time theis level of spending was unprecedented. Second, they introduced the idea of slippy maps. No longer did users need to wait for a map to load. Maps were tiled and provided a seamless experience. Third they took advantage of AJAX and rich Internet applications (RIA). Google maps was more like a desktop experience. Fourth, they made their API public. Thus it became easy for anybody to add a map to their personal Web site. Lastly, they pulled together and provided in one place a range of different spatial data - roads, satellite, birds eye panoramas - all for free.
Microsoft and Yahoo soon followed Google into the geospatial market. Microsoft have become known for their work with 3-D, birds eye and oblique views. And, like Google, they have put considerable resources behind their geospatial offerings.
The Current Landscape
The last few years have seen dramatic changes in the geospatial landscape. What exactly do we mean by this landscape? Historically, geospatial applications were confined to the desktop and limited to trained GIS programmers and analyst. The geospatial industry has become more than just GIS. It is now part of the Web and mobile experience. Maps are everywhere. There has been a proliferation of business tools enabling the inclusion and analysis of location. Thus we are talking about changes in both the breadth and depth of geospatial. For the technical, a background in geography is no longer a prerequisite. The business professional now has a wealth of new additional tools available to help better understand customers, and organize their business.
Growth within the industry continues at an astonishing rate. Microsoft, Google, Yahoo and Mapquest continue to extend the services they are offering. ESRI, historically dominating the GIS market, have extended their range of products. Many small companies also have entered the geospatial market with more specialized niche products and services.
For both the technical and less technical it is worth exploring how this landscape is changing. The different areas which have emerged, and some of the tools now available.
The Web Dynamic
As mentioned, maps have increasingly become part of the Web experience. A range of so called consumer mapping and map mash ups have emerged. Markers have been added to maps, displaying points of interest (POI’s). Maps have also become more than just points and lines; georeferenced multimedia has been added to the mix. Flickr, for example, have made sharing georeferenced photos popular. Currently around one hundred thousand photos are uploaded to Flickr each day. This has been aided by the fact that many mobile phones now have built in cameras. Consumers, businesses and government agencies have been making use of these services.

Figure 1: The FlakeFinder Web site and example of a ski resort mash up
Desktop or browser plugs-ins like Google Earth and MSN’s Virtual Earth are now popular. These have enabled 3-D maps, views from oblique angles, street level views, flight simulators and non terrestrial views.
GeoSocial Networking
Social networking has recently become very popular; services like Twitter, Facebook and MySpace. The proliferation of mobile devices with built in GPS, has added a new platform for this form of networking. The goal of geosocial networking is to discover places, things, people and events nearby. Thus, for example, when shopping in a mall, your mobile can notify you of others in your network nearby. It can also let you know about sales or special events in real time. Geospatial companies are very active in this space. Examples include Platial which is focused on ‘who and what are nearby’ on the Web. Buzzd and Pelago use Web services like Facebook Connect to share personal networks using mobile devices.
Navigation
Route and network analysis has seen dramatic recent growth. Vehicles increasingly have built in route guide systems like Tom-Tom. These systems can audibly guide you to your destination. They are often networked, thus can provide real time information on congestion, road works and other key network data. On the Web Mapquest have added local information to the existing route and network services they offer.
Mobility
With the popularity of mobile devices, a wide range of geospatial applications have been produced. The IPhone is very notable, with a range of Google map based applications now available. Other mobile providers are following suite, most notably Nokia with its OVI launch.
Tools
The geospatial community is highly active. There many open source tools available. Open source geospatial servers include MapServer and Geoserver. OpenLayers and Modest maps are excellent libraries for building mapping interfaces, while PostGIS extends the Postgres database to be able to handle spatial data.
Rich Internet mapping applications (RIA’s) are an important part of the new geospatial experience. With the adoption of technologies like AJAX and Adobe Flex, increasingly Web applications resemble those running on the desktop. Sophisticated interfaces, which avoid full screen refreshes, are ever more common. Providing users a rich Web geospatial experience.
Data
There has been a proliferation of spatial data sources. Citizens have increasingly become important as providers of this data. During Hurricane Katrina, key location information was provided and mapped by citizens. OpenStreetMap is a highly accurate free base map, built by volunteers. The proliferation of video cameras and sensors, tracking things like local weather conditions, are providing much new data to geospatial systems.

Figure 2: OpenStreetMap data displayed using OpenLayers
Business Intelligence
So how should business professionals be thinking about “geo”? We have discussed the new tools and data now available, but not how these are being directly utilized by businesses.
GIS
From the recent ESRI Business conference GIS summit a number of key business GIS areas were defined:
a) Asset Management
- Oil and Gas - monitoring and managing pipelines
b) Planning & Analysis
- Retail - market analysis and optimizing store distribution; eliminate poor performers and adding new locations.
- Insurance – evaluating risk and determining accurate pricings for the specific location.
- Emergency Services - damage assessment (eg. flooding)
- Land - land developers assessing parcel/land value
c) Field Mobility
- Trucking and delivery - optimum routes and delivery vehicle management.
d) Operational Awareness - asset utilization and supply chain efficiency.
Mash ups
There are a wide range of examples in this category. These include:
- State promotion - geolocated photos, video and images used to promote a state.
- Retail advertising - adding store locations to maps using markers.
- Real estate – homes for sale eg. http://snapshot.trulia.com/.
- Travel/tourism - destination guide and planning tools eg. http://www.flexmappers.com/videomaps
- Emergency services - geolocated data and photos eg. hurricane Katrina
- Business intelligence - news by location http://www.metacarta .com
- Financial sector - location based news and data.
- Insurance - weather forecasting eg. http://www.weather.com
- Entertainment - book or band tours eg. http://www.thetourmap.com/
- Emergency planning - toxic spills
- Health and medicine - tracking the spread of disease eg. http://www.healthmap.org/swineflu

Figure 3 – Mapping the diffusion of swine flu
- Travel industry planning – flight data eg. http://maker.geocommons.com/maps/4884
Mobile
This includes stand-alone maps or geodata downloaded from the Web
- Travel/Tourism and Federal State Agencies - local transportation information
- Trucking - fleet management
- Planning - parking information; availability by pricing zones
- Travel/Tourism - guided walks, creating routes eg. http://unitedmaps.net
- Travel - directions, traffic congestion eg. http://www.tomtom.com
- Retail - shopping alerts eg. http://www.nearbynow.com/
- Emergency services - evacuation and disaster management
- Retail – guiding drivers to low cost gasoline stations eg. http://www.dash.net
Navigation
Web based driving and route mapping services.
- Delivery - shortest path eg. http:/www.mapquest.com
- Sales – planning client visits
Geosocial Networking
- Retail advertising - are friends in my network nearby?
- Non profit - sharing ideas/creating community by locations
- Hazards and Disaster Planning – sharing information by location eg. http://en.wikipedia.org/wiki/Collaborative_mapping
- Promotion - geotagged imagery or video eg. http://www.flickr.com

Figure 4 – Geotagged photos mapped in flickr
Other
- Promotion/announcement – location driven announcements using geocoded addresses
- Publishing – interactive flash maps to support articles eg. http://www.guardian.co.uk/flash/0,,1807749,00.html
- Local advertising – finding user locations from computer IP eg. http://www.hostip.info/
Summary
Much of the current business focus is on the why and when of customer behavior; the where remains under explored. Location intelligence is the analysis of the space in which a business operates. It takes in sales, marketing, the supply chain, operations. Maps are the presentation of this spatial data, maybe in combination with spreadsheets and charts. They serve to help both with pattern recognition and as spatial indexes; displaying related data.The recent changes in the geospatial industry has provided additional tools, and data to businesses. Allowing where to be included as a part of business intelligence. In this article, we have focused on the changing geospatial landscape. We have attempted to demystify a rapidly growing industry. And, hopefully demonstrate the importance of location intelligence in the success of any business.
We are in the midst of a geospatial revolution. Geo what I hear you saying? Is this geography; maybe maps? Both and more. Specifically we are talking about location. Location in space; how it is visualized and analyzed. In this article we will introduce the new landscape that is geospatial. We will see how we got to today, define terms, compare platforms and segments. Ultimately, we will help you better understand location and its importance to your business.
Let's start at the Beginning
So what is location? At its most basic we are talking about a point in space; you, me, a house, a store, a sale, maybe a delivery. All entities and events have a location. Every business operates in this space.
So we are talking maps then. Yes and no. Maps are simply a way to visualize space. They have context. So driving maps, show roads and settlements. On thematic maps voting patterns across the US may be represented. Changes in city boundaries over time can be shown on temporal maps. More accurately, we are talking about elements or activities which exist or take place respectively in space. A map is merely the representation of these elements or activities, together or in isolation, in space. Maps are images. Humans are wired to best understand information when it is presented in images. Thus maps are a wonderful way to present complex information in understandable ways.
What is Geospatial
Geo is a prefix for any word dealing in some way with the earth. Spatial is from the word space or the boundless, three-dimensional extent in which objects and events occur with relative position and direction. Geospatial is the visualization and analysis of terrestrial geographic datasets. If “geo” is so important to understanding physical assets and the location of people - two critical components to the commerce of goods and services, and therefore business decisions - why has location been an untapped dimension of business? Why has GIS been in the closet for 25 years? And, so why suddenly has its profile been raised? A brief history might be in order.
Proprietary Software v. Spatial Thinking
Geospatial has been dominated by geographic information systems (GIS) for 25 years. GIS was a specialized niche. Desktop applications like ESRI’s ARC/INFO and ArcView allowed users to display points, lines and polygons stored in spatially enabled databases as maps, and to perform spatial analysis on this data. Thus if a store wished to build a new branch it could use a GIS to analyze proposed areas in terms of maybe demographics; we want a high percentage of families with a certain household income within a 5 mile radius of our new store.
The Role of Money
In 1996 Mapquest became the first mapping Internet site, enabling users to turn to the Web for driving directions. ESRI released its IMS product line; MapObjects IMS and ArcView IMS, in 1997. Giving developers tools to build online GIS applications. Though these were key developments it was not until 2005 with the launch of Google Maps that the geospatial revolution truly began. So why was Googles entry into geospatial so impactful? For a number of reasons. First was the amount of money put behind the launch. Take hardware for example, it is thought Google now stores many thousands of terrabytes of spatial data. At the time theis level of spending was unprecedented. Second, they introduced the idea of slippy maps. No longer did users need to wait for a map to load. Maps were tiled and provided a seamless experience. Third they took advantage of AJAX and rich Internet applications (RIA). Google maps was more like a desktop experience. Fourth, they made their API public. Thus it became easy for anybody to add a map to their personal Web site. Lastly, they pulled together and provided in one place a range of different spatial data - roads, satellite, birds eye panoramas - all for free.
Microsoft and Yahoo soon followed Google into the geospatial market. Microsoft have become known for their work with 3-D, birds eye and oblique views. And, like Google, they have put considerable resources behind their geospatial offerings.
The Current Landscape
The last few years have seen dramatic changes in the geospatial landscape. What exactly do we mean by this landscape? Historically, geospatial applications were confined to the desktop and limited to trained GIS programmers and analyst. The geospatial industry has become more than just GIS. It is now part of the Web and mobile experience. Maps are everywhere. There has been a proliferation of business tools enabling the inclusion and analysis of location. Thus we are talking about changes in both the breadth and depth of geospatial. For the technical, a background in geography is no longer a prerequisite. The business professional now has a wealth of new additional tools available to help better understand customers, and organize their business.
Growth within the industry continues at an astonishing rate. Microsoft, Google, Yahoo and Mapquest continue to extend the services they are offering. ESRI, historically dominating the GIS market, have extended their range of products. Many small companies also have entered the geospatial market with more specialized niche products and services.
For both the technical and less technical it is worth exploring how this landscape is changing. The different areas which have emerged, and some of the tools now available.
The Web Dynamic
As mentioned, maps have increasingly become part of the Web experience. A range of so called consumer mapping and map mash ups have emerged. Markers have been added to maps, displaying points of interest (POI’s). Maps have also become more than just points and lines; georeferenced multimedia has been added to the mix. Flickr, for example, have made sharing georeferenced photos popular. Currently around one hundred thousand photos are uploaded to Flickr each day. This has been aided by the fact that many mobile phones now have built in cameras. Consumers, businesses and government agencies have been making use of these services.

Figure 1: The FlakeFinder Web site and example of a ski resort mash up
Desktop or browser plugs-ins like Google Earth and MSN’s Virtual Earth are now popular. These have enabled 3-D maps, views from oblique angles, street level views, flight simulators and non terrestrial views.
GeoSocial Networking
Social networking has recently become very popular; services like Twitter, Facebook and MySpace. The proliferation of mobile devices with built in GPS, has added a new platform for this form of networking. The goal of geosocial networking is to discover places, things, people and events nearby. Thus, for example, when shopping in a mall, your mobile can notify you of others in your network nearby. It can also let you know about sales or special events in real time. Geospatial companies are very active in this space. Examples include Platial which is focused on ‘who and what are nearby’ on the Web. Buzzd and Pelago use Web services like Facebook Connect to share personal networks using mobile devices.
Navigation
Route and network analysis has seen dramatic recent growth. Vehicles increasingly have built in route guide systems like Tom-Tom. These systems can audibly guide you to your destination. They are often networked, thus can provide real time information on congestion, road works and other key network data. On the Web Mapquest have added local information to the existing route and network services they offer.
Mobility
With the popularity of mobile devices, a wide range of geospatial applications have been produced. The IPhone is very notable, with a range of Google map based applications now available. Other mobile providers are following suite, most notably Nokia with its OVI launch.
Tools
The geospatial community is highly active. There many open source tools available. Open source geospatial servers include MapServer and Geoserver. OpenLayers and Modest maps are excellent libraries for building mapping interfaces, while PostGIS extends the Postgres database to be able to handle spatial data.
Rich Internet mapping applications (RIA’s) are an important part of the new geospatial experience. With the adoption of technologies like AJAX and Adobe Flex, increasingly Web applications resemble those running on the desktop. Sophisticated interfaces, which avoid full screen refreshes, are ever more common. Providing users a rich Web geospatial experience.
Data
There has been a proliferation of spatial data sources. Citizens have increasingly become important as providers of this data. During Hurricane Katrina, key location information was provided and mapped by citizens. OpenStreetMap is a highly accurate free base map, built by volunteers. The proliferation of video cameras and sensors, tracking things like local weather conditions, are providing much new data to geospatial systems.

Figure 2: OpenStreetMap data displayed using OpenLayers
Business Intelligence
So how should business professionals be thinking about “geo”? We have discussed the new tools and data now available, but not how these are being directly utilized by businesses.
GIS
From the recent ESRI Business conference GIS summit a number of key business GIS areas were defined:
a) Asset Management
- Oil and Gas - monitoring and managing pipelines
b) Planning & Analysis
- Retail - market analysis and optimizing store distribution; eliminate poor performers and adding new locations.
- Insurance – evaluating risk and determining accurate pricings for the specific location.
- Emergency Services - damage assessment (eg. flooding)
- Land - land developers assessing parcel/land value
c) Field Mobility
- Trucking and delivery - optimum routes and delivery vehicle management.
d) Operational Awareness - asset utilization and supply chain efficiency.
Mash ups
There are a wide range of examples in this category. These include:
- State promotion - geolocated photos, video and images used to promote a state.
- Retail advertising - adding store locations to maps using markers.
- Real estate – homes for sale eg. http://snapshot.trulia.com/.
- Travel/tourism - destination guide and planning tools eg. http://www.flexmappers.com/videomaps
- Emergency services - geolocated data and photos eg. hurricane Katrina
- Business intelligence - news by location http://www.metacarta .com
- Financial sector - location based news and data.
- Insurance - weather forecasting eg. http://www.weather.com
- Entertainment - book or band tours eg. http://www.thetourmap.com/
- Emergency planning - toxic spills
- Health and medicine - tracking the spread of disease eg. http://www.healthmap.org/swineflu

Figure 3 – Mapping the diffusion of swine flu
- Travel industry planning – flight data eg. http://maker.geocommons.com/maps/4884
Mobile
This includes stand-alone maps or geodata downloaded from the Web
- Travel/Tourism and Federal State Agencies - local transportation information
- Trucking - fleet management
- Planning - parking information; availability by pricing zones
- Travel/Tourism - guided walks, creating routes eg. http://unitedmaps.net
- Travel - directions, traffic congestion eg. http://www.tomtom.com
- Retail - shopping alerts eg. http://www.nearbynow.com/
- Emergency services - evacuation and disaster management
- Retail – guiding drivers to low cost gasoline stations eg. http://www.dash.net
Navigation
Web based driving and route mapping services.
- Delivery - shortest path eg. http:/www.mapquest.com
- Sales – planning client visits
Geosocial Networking
- Retail advertising - are friends in my network nearby?
- Non profit - sharing ideas/creating community by locations
- Hazards and Disaster Planning – sharing information by location eg. http://en.wikipedia.org/wiki/Collaborative_mapping
- Promotion - geotagged imagery or video eg. http://www.flickr.com

Figure 4 – Geotagged photos mapped in flickr
Other
- Promotion/announcement – location driven announcements using geocoded addresses
- Publishing – interactive flash maps to support articles eg. http://www.guardian.co.uk/flash/0,,1807749,00.html
- Local advertising – finding user locations from computer IP eg. http://www.hostip.info/
Summary
Much of the current business focus is on the why and when of customer behavior; the where remains under explored. Location intelligence is the analysis of the space in which a business operates. It takes in sales, marketing, the supply chain, operations. Maps are the presentation of this spatial data, maybe in combination with spreadsheets and charts. They serve to help both with pattern recognition and as spatial indexes; displaying related data.The recent changes in the geospatial industry has provided additional tools, and data to businesses. Allowing where to be included as a part of business intelligence. In this article, we have focused on the changing geospatial landscape. We have attempted to demystify a rapidly growing industry. And, hopefully demonstrate the importance of location intelligence in the success of any business.
Twitter and Contracting
I follow Jesse wardens blog. He is an ace Flash and Flex developer. Though not an RIA map developer, he has lots of useful tips and tricks on general RIA development. I particularly liked his most recent entry on contracting and twitter
Wednesday, July 15, 2009
Mapping and MVC
I found here a nice article on the Model-View-Control design architecture/pattern. Its a useful pattern to know about and this article lays things out nicely.
Geospatial Thoughts
I started out as a Physical Geographer. The early days of the Internet coincided with my move from England to the US. The possibilities of a geospatial Web led me to GIS in the mid 1990's. I took a Masters degree and dipped my toe into geospatial programming. Over 10 years later I'm still programming. The early geospatial efforts I worked with - MapObjects IMS, ArcIMS and Viewers - were a start. But somewhat unfulfilling for a user; waiting for new map images to reload after pans and zooms was a pain. I always wondered about how the user experience could be improved. This led me to Flash and now Flex or rich internet mapping applications.
My working life is spent surrounded by computer scientists. But, I remain first a geographer second a programmer. People have asked me what that means. I usually reply that I think about my work geospatially. "You mean in terms of maps?" is often the response. It struck me, this is how many think of geography and geospatial.
I'm writing an article for the new LBx Journal. Its aimed at business executives. I'll post it on the blog when I am done. It deals in more depth with this issue. This may form the first in a series exploring geospatial and business. At a basic level how can business take advantage of geospatial? Increasingly I view my work from a business angle. The geospatial community seems focused on the 'building and they will come' approach. If geospatial becomes an integrated part of business, and not separate as it is today, we need to start demonstrating its power to solve business problems. I attend too many geospatial conferences where 'how can we make money' is a central topic. Let's start looking through the lens of a business executive. Understand their perspective, then take our knowledge of geospatial and provide them with solutions. Focus on understanding the problem and monetizing solutions.
My working life is spent surrounded by computer scientists. But, I remain first a geographer second a programmer. People have asked me what that means. I usually reply that I think about my work geospatially. "You mean in terms of maps?" is often the response. It struck me, this is how many think of geography and geospatial.
I'm writing an article for the new LBx Journal. Its aimed at business executives. I'll post it on the blog when I am done. It deals in more depth with this issue. This may form the first in a series exploring geospatial and business. At a basic level how can business take advantage of geospatial? Increasingly I view my work from a business angle. The geospatial community seems focused on the 'building and they will come' approach. If geospatial becomes an integrated part of business, and not separate as it is today, we need to start demonstrating its power to solve business problems. I attend too many geospatial conferences where 'how can we make money' is a central topic. Let's start looking through the lens of a business executive. Understand their perspective, then take our knowledge of geospatial and provide them with solutions. Focus on understanding the problem and monetizing solutions.
Labels:
business geographics,
geospatial,
problem solving
Mapping and Sensed Location
Thinking about geospatial, many people focus only on maps. But maps are simply a tool to visualise spatial data. I've recently become interested in sensed locations, or knowing where a user is from GPS or IP geolocation. The latter is fascinating. Taking a best guess at where a user is based on their IP address. There is more on this at the following slideshow
Though there are obviously privacy issues here. Knowing, for example, where a user is when accessing your web site, can help tune the experience. Maybe allowing developers to provide more relevant content.
Local data and search are my current areas of focus. This is very relevant. More on this in subsequent posts.
Though there are obviously privacy issues here. Knowing, for example, where a user is when accessing your web site, can help tune the experience. Maybe allowing developers to provide more relevant content.
Local data and search are my current areas of focus. This is very relevant. More on this in subsequent posts.
Thursday, July 2, 2009
Everyblock Code Open Sourced
The source code for Everyblock has been open sourced. You can now access all source code . It is a nice application. Their dilemma has been much discussed; how to make money when the code is required to be open sourced. Reading the blurb in the code release page, one feels there is some degree of resentment about having to go open source. One has to sympathise, but when they agreed on their financing they knew this to be the arrangement. Its odd they did not plan on this when they built their business plan.
Flash CS3 Map Evolution

The Flash CS maps I have been building using AS3 is moving along slowly. I shared a link in an earlier blog entry to the first phase. Most of the time here was spent assembling the assets ie. movieclips of each continent/country/state and supporting XML. The XML is tied to the movieclip via a number - <id>1</id>, sprite 1. Thus I have an all continents swf and individual continents eg. northamerica.swf. These are made up of individual country/state movieclips. Clicking on a continent, loads the individual continent swf. Since Flex is the glue for the swf's, I have used eventing to communicate between each of the swf's; flash to flex, flex to flash. The eventing code I used was an adaptation of code shared by Jason Fincanon. The code is as follows:
The next phase of the map I have also now posted.

Here I have integrated the zoom functionality from Peter Organa (see earlier post). Thus the zoom.swf provided by Peter now listens to which continent was selected and loads the appropriate swf. Now a selected continent can be panned, zoomed into and out. This will allow, in the next phase, geolocated pins to be added to the map.
Labels:
0,
actionscript 3,
AS3,
consumer maps,
Flash CS3,
maps
Wednesday, July 1, 2009
ArcGIS and the IPhone
I took the following from James Fee's blog:
Q: Will ESRI support the iPhone?
Yes, we will support the iPhone as a mobile platform to get maps from ArcGIS Server and do queries and edits on data from ArcGIS Server. We plan on releasing an application for the iPhone later this year and then adding additional capability as part of our 9.4 release. In addition developers can build their own solutions for the iPhone using the REST APIs available from ArcGIS Server.
I didn't think this would be too far away.
On another mobile note. I'm in line for beta testing the Nokia OVI API. Its an interesting process. Nokia have a limited number of beta slots. Once considered you then need to explain the mobile application you plan to build and whether you can get it done in 2 months.
Q: Will ESRI support the iPhone?
Yes, we will support the iPhone as a mobile platform to get maps from ArcGIS Server and do queries and edits on data from ArcGIS Server. We plan on releasing an application for the iPhone later this year and then adding additional capability as part of our 9.4 release. In addition developers can build their own solutions for the iPhone using the REST APIs available from ArcGIS Server.
I didn't think this would be too far away.
On another mobile note. I'm in line for beta testing the Nokia OVI API. Its an interesting process. Nokia have a limited number of beta slots. Once considered you then need to explain the mobile application you plan to build and whether you can get it done in 2 months.
Saturday, June 27, 2009
Flash Transitions
Friday, June 26, 2009
Flash Map Convert lat/long to x,y
I need to add pins to the flash map I'm working on. They are geo-located based on lat/long stored in a loaded XML. I was wondering how to do the conversion from lat/long to screen x,y. Found this thread. I'll give feedback on the solution I finally implemented in due course.
Labels:
AS3,
Flash CS3,
lat/long conversion to screen x,
y
Flash CS3 Map Zoom
I've been building a Flash CS3 map mentioned earlier in the blog. The next thing to be done was zoom. From past experience this is always a tricky business. I wondered if anybody might have shared their solution. Eureka! Peter Organa to the rescue. He has written just the best zoom and pan tool. Scroll wheel even works. Best of all its in AS3 and Peter has shared the source. Made my day!
Labels:
actionscript 3,
consumer mapping,
Flash CS3,
map zoom tool
Thursday, June 25, 2009
HTML 5
I came across this discussion on James Fees blog about HTML 5. So is there a future for Flash and Silverlight? I'm inclined to think so. The great thing is more ways to develop RIA. Here is a link to the article.
Flash Builder 4
The full release of Flash Builder 4 is scheduled sometime in July, replacing Flex Builder 3. I thought I'd take a look at what is different. Using the Modest Maps API I built a demo mapping application. I thought I'd post a brief snippet of that code. The first is the code in Flex 3:
<?xml version="1.0" encoding="utf-8"?>
<mx:Application xmlns:mx="http://www.adobe.com/2006/mxml"
minWidth="1024" minHeight="768" creationComplete="init()">
<mx:Script>
<![CDATA[
import mx.core.UIComponent;
import com.modestmaps.TweenMap;
import com.modestmaps.mapproviders.OpenStreetMapProvider;
import com.modestmaps.geo.Location;
private function init():void
{
}
]]>
</mx:Script>
<mx:Canvas id="mappanel" width="100%" height="97%"/>
</mx:Application>
This second is the same code using Flash Builder 4:
<?xml version="1.0" encoding="utf-8"?>
<s:Application xmlns:fx="http://ns.adobe.com/mxml/2009" xmlns:s="library://ns.adobe.com/flex/spark" xmlns:mx="library://ns.adobe.com/flex/halo"
minWidth="1024" minHeight="768" creationComplete="init()">
<fx:Script>
<![CDATA[
import mx.core.UIComponent;
import com.modestmaps.TweenMap;
import com.modestmaps.mapproviders.OpenStreetMapProvider;
import com.modestmaps.geo.Location;
private function init():void
{
}
]]>
</fx:Script>
<mx:Canvas id="mappanel" width="100%" height="97%"/>
</s:Application>
There are some interesting differences. I had expected to be able simply to extend my existing Flex 3 apps. Not so. I'll blog more on this when I have more time to poke around.
<?xml version="1.0" encoding="utf-8"?>
<mx:Application xmlns:mx="http://www.adobe.com/2006/mxml"
minWidth="1024" minHeight="768" creationComplete="init()">
<mx:Script>
<![CDATA[
import mx.core.UIComponent;
import com.modestmaps.TweenMap;
import com.modestmaps.mapproviders.OpenStreetMapProvider;
import com.modestmaps.geo.Location;
private function init():void
{
}
]]>
</mx:Script>
<mx:Canvas id="mappanel" width="100%" height="97%"/>
</mx:Application>
This second is the same code using Flash Builder 4:
<?xml version="1.0" encoding="utf-8"?>
<s:Application xmlns:fx="http://ns.adobe.com/mxml/2009" xmlns:s="library://ns.adobe.com/flex/spark" xmlns:mx="library://ns.adobe.com/flex/halo"
minWidth="1024" minHeight="768" creationComplete="init()">
<fx:Script>
<![CDATA[
import mx.core.UIComponent;
import com.modestmaps.TweenMap;
import com.modestmaps.mapproviders.OpenStreetMapProvider;
import com.modestmaps.geo.Location;
private function init():void
{
}
]]>
</fx:Script>
<mx:Canvas id="mappanel" width="100%" height="97%"/>
</s:Application>
There are some interesting differences. I had expected to be able simply to extend my existing Flex 3 apps. Not so. I'll blog more on this when I have more time to poke around.
Geo-Spatial Solutions in Challenging Economic Times
Introduction
The landscape of the geo-spatial industry is undergoing rapid changes. Some suggest a geo-revolution is underway. The range of products and platforms is broadening. Existing boundaries are blurring. In parallel, the world is experiencing an unprecedented economic downturn. Start-ups and established businesses are increasingly looking for low cost solutions. This article will look at the current state of the geo-spatial industry. It will discuss some of the software and base map sources given tightening budgets.
Is Google Maps GIS?
Google maps was launched in November 2005. Thus began the rapid expansion of the geo-industry. Yahoo and Microsoft soon entered the market. Mapquest extended its offering and ESRI broadened their business. All made public their mapping API’s. Slippy tiled base maps became easy to add to any web sites. But are these consumer maps, often described as mash ups, GIS? This has been much discussed on the Web (http://highearthorbit.com/is-googlemaps-gis/). Though there are many differing opinions, the general agreement is that the boundaries are rapidly blurring. It might be worth for this discussion, to include these new, currently free tools.
The GIS Stack
The classic GIS stack is usually discussed in terms of the client, server and database. We will add an additional category, that of tools for tiling and caching.
Client
Increasingly geo-companies are focused on building rich Internet mapping applications (RIA). That is, applications which more closely resemble those running on the desktop. Two technologies dominate; those applications which run in the Flash player, and those built using AJAX. The Flash player is an Adobe product, which runs on over 90% of all Web browsers. Applications which run in the player can be written in Adobe Flash or Flex using the Actionscript programming language. Actionscript 3.0 (AS3) is the current version, and the one discussed here. The Flash and Flex SDK’s are free, though their respective IDE’s will need purchasing. AJAX is built on Javascript. It is completely open source, and supported by a large user community. One more RIA technology is worth mentioning. Microsoft recently released Silverlight, in direct competition with Flex. Like Flex, the SDK is free, but not the Visual Studio IDE.
Figure 1 – Some of the wide array of options for building an open source GIS stack
The first two options in the client layer we will discuss are pure open source. They are both the most accessible and flexible solutions. OpenLayers (http://openlayers.org) was developed by MetaCarta (http://www.metacarta.com/) and is a Javascript library. Modest Maps is an AS3 mapping library. Developed in part by Stamen Design (http://stamen.com/), Modest Maps has a sizeable user community, which provides excellent support. Both of these solutions provide full access to the base code. This allows developers to customize at their own discretion. In each case, these clients can access a wide range of different base maps.
Figure 2 – FlakeFinder a ski resort mash up using Modest Maps
Less open but still free are the mapping API’s from Google, Microsoft (MSN), Yahoo, Mapquest and ESRI. They break down as follows:
Google API’s – (http://code.google.com/apis/maps/documentation/index.html) - AS3, Javascript
MSN API’s – AJAX, .NET
Mapquest API’s (http://developer.mapquest.com/) – AS3, Javascript, C++, Java, .NET
Yahoo API’s (http://developer.yahoo.com/maps/) – AJAX, AS3
ESRI API’s (http://resources.esri.com/arcgisserver/index.cfm?fa=applications) – AS3, Silverlight, .NET, Java
The use of each API requires a free key. They provide a range of services including marker overlay, routing, styling and geocoding, The ESRI API’s provide the broadest range of GIS tools. They can also access many different map services including Google, MSN and the GeoWebCache/Geoserver and TileCache/MapServer combinations. Recently added to the ESRI offering is an API for Silverlight.
Tiling and Caching
Geoserver will generate tiles for maps automatically, or use seeding to pre-generate tiles, Mapserver relies on ka-Map for tile generation and viewing.
There are two main caching solutions. Each dramatically improves the performance of any mapping application. TileCache (http://tilecache.org/) is another MetaCarta open source product. It is Python based and supports multiple different rendering backends, most notably MapServer. GeoWebCache (http://geowebcache.org/trac) is written in Java. It caches map tiles as they are requested, in effect acting as a proxy between client and server. Based on the request, if no pre-rendered tiles are found, it calls the server to render new tiles. GeoWebCache works with GeoServer, or any WMS-compliant server.
Map Servers
There are many map server solutions. But, two low cost solutions stand out. First there is MapServer (http://mapserver.org/). This is a very popular open source solution. It runs under the Apache Web Server and is supported by an excellent PHP based scripting language called Mapscript. MapServer is a Web map rendering engine. GeoServer (http://geoserver.org) is the second open source spatial server. Built in Java, it is a complete spatial Web environment, often classified both as a rendering engine and application framework.
MapServer and Geoserver can be integrated easily with maps from Google, MSN and Yahoo. They both compare favorably in terms of performance. Geoserver comes with a management interface, this makes set up and configuration somewhat easier than for MapServer. But the lack of need for an application server and the excellent Mapscript, are among the many attractions of MapServer.
Figure 3 – MarineMap is an RIA which uses OpenLayers and Google base maps.
Databases
Arguably, the most robust spatial open source database solution is the PostGIS/Postgres combination. PostGIS was developed by Paul Ramsey. He provides excellent documentation and support via his blog (http://blog.cleverelephant.ca/). PostGIS is an extension of Postgres. Like ESRI’s ArcSDE is allows relational databases to work with spatial data. Technically though they are quite different, PostGIS is part of the database while ArcSDE is spatial middleware, running as a separate process.
Mobile Platform
The majority of mobile mapping applications currently run on the IPhone. Most have been built using the Google maps Javascript (version 3) API. Maps have been much the focus in the mobile space recently. Nokia have just launched OVI Maps and are in the process of exposing the Javascript API to developers. The AS2 version of Modest Maps allows Flash Lite development, which will run on any handset with the Flash player installed.
Summary
There are many low cost solutions available for building geo-spatial applications. This article walked through the GIS stack, discussing some of these options. Increasingly, as budgets tighten, companies are turning to these open source development tools. Often they are surprised by what they find.
References
http://geoserver.org/display/GEOSDOC/Google+Maps - Geoserver and Google maps integration
http://www.rvaidya.com/blog/gis/2009/02/12/mapserver-using-google-maps-with-php-mapscript/ - MapServer and Google maps integration
http://kelsocartography.com/blog/?p=2257 - Marine Maps discussion
http://spatialhorizons.com/2007/11/14/using-mapserver-2-generating-map-tiles/ - generating map tiles for MapServer using ka-Map.
The landscape of the geo-spatial industry is undergoing rapid changes. Some suggest a geo-revolution is underway. The range of products and platforms is broadening. Existing boundaries are blurring. In parallel, the world is experiencing an unprecedented economic downturn. Start-ups and established businesses are increasingly looking for low cost solutions. This article will look at the current state of the geo-spatial industry. It will discuss some of the software and base map sources given tightening budgets.
Is Google Maps GIS?
Google maps was launched in November 2005. Thus began the rapid expansion of the geo-industry. Yahoo and Microsoft soon entered the market. Mapquest extended its offering and ESRI broadened their business. All made public their mapping API’s. Slippy tiled base maps became easy to add to any web sites. But are these consumer maps, often described as mash ups, GIS? This has been much discussed on the Web (http://highearthorbit.com/is-googlemaps-gis/). Though there are many differing opinions, the general agreement is that the boundaries are rapidly blurring. It might be worth for this discussion, to include these new, currently free tools.
The GIS Stack
The classic GIS stack is usually discussed in terms of the client, server and database. We will add an additional category, that of tools for tiling and caching.
Client
Increasingly geo-companies are focused on building rich Internet mapping applications (RIA). That is, applications which more closely resemble those running on the desktop. Two technologies dominate; those applications which run in the Flash player, and those built using AJAX. The Flash player is an Adobe product, which runs on over 90% of all Web browsers. Applications which run in the player can be written in Adobe Flash or Flex using the Actionscript programming language. Actionscript 3.0 (AS3) is the current version, and the one discussed here. The Flash and Flex SDK’s are free, though their respective IDE’s will need purchasing. AJAX is built on Javascript. It is completely open source, and supported by a large user community. One more RIA technology is worth mentioning. Microsoft recently released Silverlight, in direct competition with Flex. Like Flex, the SDK is free, but not the Visual Studio IDE.

Figure 1 – Some of the wide array of options for building an open source GIS stack
The first two options in the client layer we will discuss are pure open source. They are both the most accessible and flexible solutions. OpenLayers (http://openlayers.org) was developed by MetaCarta (http://www.metacarta.com/) and is a Javascript library. Modest Maps is an AS3 mapping library. Developed in part by Stamen Design (http://stamen.com/), Modest Maps has a sizeable user community, which provides excellent support. Both of these solutions provide full access to the base code. This allows developers to customize at their own discretion. In each case, these clients can access a wide range of different base maps.

Figure 2 – FlakeFinder a ski resort mash up using Modest Maps
Less open but still free are the mapping API’s from Google, Microsoft (MSN), Yahoo, Mapquest and ESRI. They break down as follows:
Google API’s – (http://code.google.com/apis/maps/documentation/index.html) - AS3, Javascript
MSN API’s – AJAX, .NET
Mapquest API’s (http://developer.mapquest.com/) – AS3, Javascript, C++, Java, .NET
Yahoo API’s (http://developer.yahoo.com/maps/) – AJAX, AS3
ESRI API’s (http://resources.esri.com/arcgisserver/index.cfm?fa=applications) – AS3, Silverlight, .NET, Java
The use of each API requires a free key. They provide a range of services including marker overlay, routing, styling and geocoding, The ESRI API’s provide the broadest range of GIS tools. They can also access many different map services including Google, MSN and the GeoWebCache/Geoserver and TileCache/MapServer combinations. Recently added to the ESRI offering is an API for Silverlight.
Tiling and Caching
Geoserver will generate tiles for maps automatically, or use seeding to pre-generate tiles, Mapserver relies on ka-Map for tile generation and viewing.
There are two main caching solutions. Each dramatically improves the performance of any mapping application. TileCache (http://tilecache.org/) is another MetaCarta open source product. It is Python based and supports multiple different rendering backends, most notably MapServer. GeoWebCache (http://geowebcache.org/trac) is written in Java. It caches map tiles as they are requested, in effect acting as a proxy between client and server. Based on the request, if no pre-rendered tiles are found, it calls the server to render new tiles. GeoWebCache works with GeoServer, or any WMS-compliant server.
Map Servers
There are many map server solutions. But, two low cost solutions stand out. First there is MapServer (http://mapserver.org/). This is a very popular open source solution. It runs under the Apache Web Server and is supported by an excellent PHP based scripting language called Mapscript. MapServer is a Web map rendering engine. GeoServer (http://geoserver.org) is the second open source spatial server. Built in Java, it is a complete spatial Web environment, often classified both as a rendering engine and application framework.
MapServer and Geoserver can be integrated easily with maps from Google, MSN and Yahoo. They both compare favorably in terms of performance. Geoserver comes with a management interface, this makes set up and configuration somewhat easier than for MapServer. But the lack of need for an application server and the excellent Mapscript, are among the many attractions of MapServer.

Figure 3 – MarineMap is an RIA which uses OpenLayers and Google base maps.
Databases
Arguably, the most robust spatial open source database solution is the PostGIS/Postgres combination. PostGIS was developed by Paul Ramsey. He provides excellent documentation and support via his blog (http://blog.cleverelephant.ca/). PostGIS is an extension of Postgres. Like ESRI’s ArcSDE is allows relational databases to work with spatial data. Technically though they are quite different, PostGIS is part of the database while ArcSDE is spatial middleware, running as a separate process.
Mobile Platform
The majority of mobile mapping applications currently run on the IPhone. Most have been built using the Google maps Javascript (version 3) API. Maps have been much the focus in the mobile space recently. Nokia have just launched OVI Maps and are in the process of exposing the Javascript API to developers. The AS2 version of Modest Maps allows Flash Lite development, which will run on any handset with the Flash player installed.
Summary
There are many low cost solutions available for building geo-spatial applications. This article walked through the GIS stack, discussing some of these options. Increasingly, as budgets tighten, companies are turning to these open source development tools. Often they are surprised by what they find.
References
http://geoserver.org/display/GEOSDOC/Google+Maps - Geoserver and Google maps integration
http://www.rvaidya.com/blog/gis/2009/02/12/mapserver-using-google-maps-with-php-mapscript/ - MapServer and Google maps integration
http://kelsocartography.com/blog/?p=2257 - Marine Maps discussion
http://spatialhorizons.com/2007/11/14/using-mapserver-2-generating-map-tiles/ - generating map tiles for MapServer using ka-Map.
MarineMap Decision Support Tool

I came across the Marine maps application while looking at the Farallon Geographics Web site. Dennis Wuthrich CEO of Farallon, was at Where 2.0, so I had a chance to ask him about it. You can link to it at MarineMap Viewer
This is a classic example of a Web 2.0 mapping application. It is so well designed, with transitions, drag and drop and combines metadata and the interactive map in such a well designed manner. I'm a huge fan. I'm writing another article, this one for gisdevelopment.net (see next post). While researching the article I came upon an interview on the development of MarineMap at the following link MarineMap Discussion. It is well worth a read.
Flash CS3 Actionscript 3 and Maps
It has been a long while since I worked with the Flash IDE. Going back to Flash MX in fact. I'm approached quite often about Flash jobs. Often they ask for Actionscript 2.0 which I often find a little odd. Must be a good reason. Anyway, I enjoy working with AS3 in Flex so thought it time to return to Flash. I mentioned in an earlier post that I was using both the Flash CS3 IDE and Flex Builder. It took a little getting used to but I'm beginning to get comfortable. I've been building a vector based Flash map application:
Flash CS3 AS3 Map
I'll admit to being rather wrapped up in it. The continents/countries/states are individual movieclips. Metadata is loaded from XML. Flex is being used to tie the main 'all continents' swf to individual continents. There are 8 swf's in all. Its a cool little app I think. Rather than building one honking big swf, break everything into smaller pieces and use Flex as the glue. I have a list of things to do. Adding zoom to the right pane, and overlaying pins (from a lat/long conversion) using loaded XML will make it a really functional application.
I've avoided mentioning animation. I'll admit i'm very interested. In AS2 I avoided it like the plague. In CS3 AS3 it seems easier. I've played somewhat and found it all pretty straighforward. I plan to add a little cool animation to this app...
Flash CS3 AS3 Map
I'll admit to being rather wrapped up in it. The continents/countries/states are individual movieclips. Metadata is loaded from XML. Flex is being used to tie the main 'all continents' swf to individual continents. There are 8 swf's in all. Its a cool little app I think. Rather than building one honking big swf, break everything into smaller pieces and use Flex as the glue. I have a list of things to do. Adding zoom to the right pane, and overlaying pins (from a lat/long conversion) using loaded XML will make it a really functional application.
I've avoided mentioning animation. I'll admit i'm very interested. In AS2 I avoided it like the plague. In CS3 AS3 it seems easier. I've played somewhat and found it all pretty straighforward. I plan to add a little cool animation to this app...
Labels:
Actionscript 2.0,
Flash CS3,
Flex custom maps,
maps
Subscribe to:
Posts (Atom)